An algorithm is a step-by-step procedure or set of rules designed to perform a specific task or solve a problem. It is a logical sequence of actions that takes an input, processes it, and produces an output.
In the context of computing, an algorithm is a finite set of instructions written in a formal language that can be executed by a machine (e.g., a computer). Algorithms are the foundation of problem-solving in programming, mathematics, and other scientific disciplines.
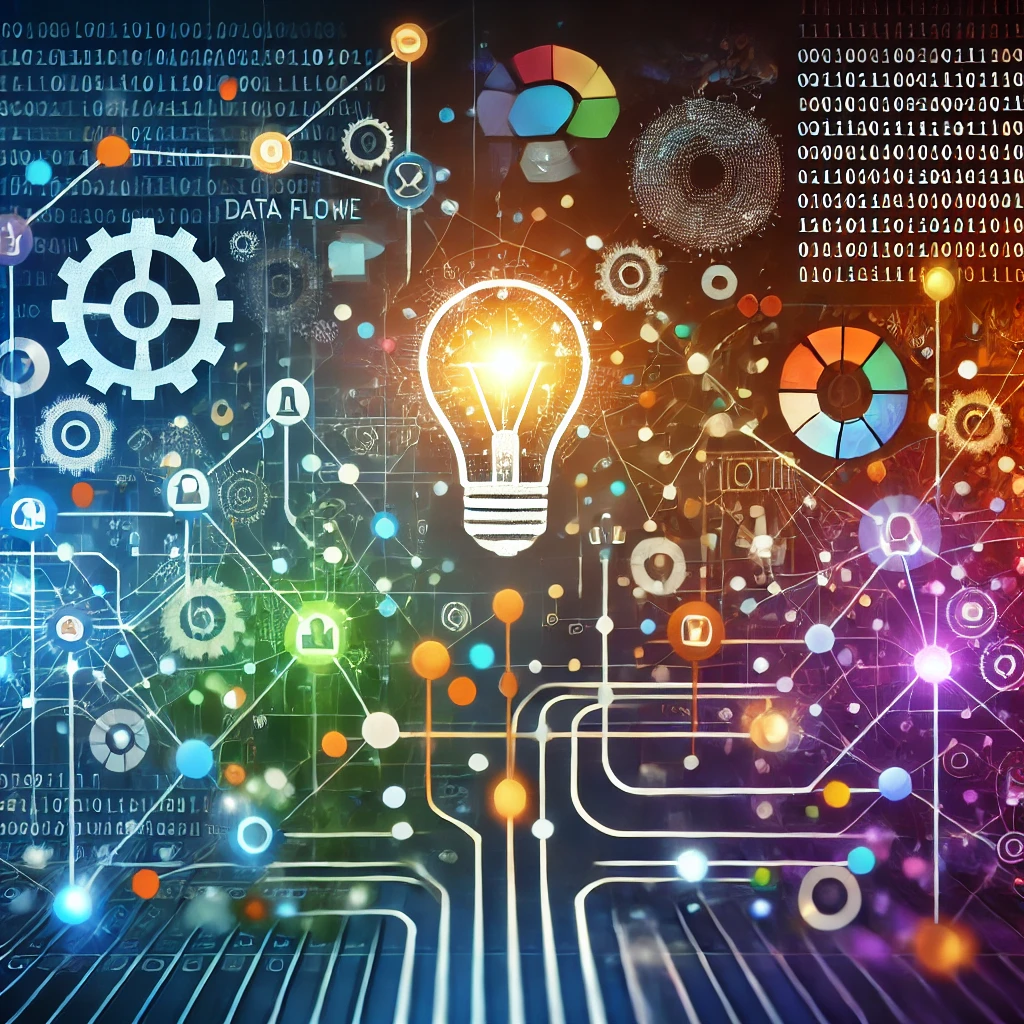
Characteristics of an Algorithm:
- Finiteness: An algorithm must have a finite number of steps.
- Definiteness: Each step must be precisely defined.
- Input: It may accept zero or more inputs.
- Output: It must produce at least one output.
- Effectiveness: Each step should be basic enough to be performed in a finite amount of time.
Importance of Algorithms
Algorithms are crucial in various fields and have a profound impact on how problems are solved efficiently and effectively. Here’s why they are important:
1. Efficiency
Algorithms help optimize resources such as time and memory.
Efficient algorithms can process large datasets or perform tasks in less time, which is critical in real-world applications (e.g., search engines, data processing).
2. Foundation of Computer Science
Algorithms form the backbone of computer science, enabling software development, artificial intelligence, cryptography, and network design.
3. Problem Solving
Algorithms provide structured approaches to solving complex problems systematically.
They break down problems into smaller, manageable steps.
4. Scalability
Good algorithms ensure scalability, enabling systems to handle increased loads or larger datasets without performance degradation.
5. Universality
Algorithms are not tied to a specific language or platform. They can be implemented in any programming language or system, making them versatile.
6. Automation
Algorithms power automation in industries by enabling machines to perform repetitive or complex tasks without human intervention (e.g., robotics, manufacturing).
Algorithm Basics
List of Most Important Algorithms
In the following, we provide an exhaustive list of all algorithms grouped by their area of application:
1 Sorting Algorithms
- Bubble Sort
- Insertion Sort
- Selection Sort
- Merge Sort
- Quick Sort
- Heap Sort
- Radix Sort
- Bucket Sort
- Counting Sort
- Tim Sort
2 Search Algorithms
- Linear Search
- Binary Search
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Jump Search
- Interpolation Search
- Exponential Search
- A* Search
- Dijkstra’s Algorithm
3 Graph Algorithms
- Kruskal’s Algorithm (Minimum Spanning Tree)
- Prim’s Algorithm (Minimum Spanning Tree)
- Bellman-Ford Algorithm
- Floyd-Warshall Algorithm
- Johnson’s Algorithm
- Tarjan’s Algorithm (Strongly Connected Components)
- Kosaraju’s Algorithm
- Topological Sort
- Edmonds-Karp Algorithm (Maximum Flow)
- Ford-Fulkerson Algorithm (Maximum Flow)
4 Dynamic Programming Algorithms
- Longest Common Subsequence (LCS)
- Longest Increasing Subsequence (LIS)
- Matrix Chain Multiplication
- Knapsack Problem
- Floyd-Warshall Algorithm (Shortest Paths)
- Bellman-Ford Algorithm (Shortest Paths)
- Edit Distance / Levenshtein Distance
- Partition Problem
- Coin Change Problem
- Rod Cutting Problem
5 Divide and Conquer Algorithms
- Merge Sort
- Quick Sort
- Binary Search
- Closest Pair of Points
- Strassen’s Matrix Multiplication
6 String Algorithms
- Knuth-Morris-Pratt (KMP) Algorithm
- Rabin-Karp Algorithm
- Z Algorithm
- Aho-Corasick Algorithm
- Boyer-Moore Algorithm
- Suffix Tree and Suffix Array
- Longest Common Prefix (LCP) Array
7 Machine Learning Algorithms
- Linear Regression
- Logistic Regression
- Decision Trees
- Random Forest
- Support Vector Machines (SVM)
- K-Nearest Neighbors (KNN)
- K-Means Clustering
- Principal Component Analysis (PCA)
- Neural Networks
- Gradient Boosting (XGBoost, LightGBM, etc.)
8 Numerical Algorithms
- Newton-Raphson Method
- Gaussian Elimination
- Jacobi Method
- Gauss-Seidel Method
- Simpson’s Rule
- Trapezoidal Rule
- Monte Carlo Method
- Fast Fourier Transform (FFT)
9 Cryptographic Algorithms
- RSA Algorithm
- Diffie-Hellman Key Exchange
- Elliptic Curve Cryptography (ECC)
- Advanced Encryption Standard (AES)
- Data Encryption Standard (DES)
- Secure Hash Algorithm (SHA)
- MD5
10 Computational Geometry Algorithms
- Convex Hull (Graham’s Scan, Jarvis March)
- Line Intersection
- Voronoi Diagram
- Delaunay Triangulation
- Closest Pair of Points
- Sweep Line Algorithm
- Bentley-Ottmann Algorithm
11 Miscellaneous Algorithms
- Backtracking Algorithms (e.g., N-Queens Problem, Sudoku Solver)
- Greedy Algorithms (e.g., Activity Selection, Huffman Coding)
- Monte Carlo Algorithms
- Simulated Annealing
- Genetic Algorithms
- Branch and Bound Algorithms
12 Distributed and Parallel Algorithms
- MapReduce
- Paxos Algorithm
- Raft Consensus Algorithm
- Bully Algorithm (Leader Election)
- Distributed Hash Tables (DHT)