Bash Arithmetic Expansion
Bash Arithmetic Expansion allows you to perform arithmetic operations within your Bash scripts. This feature simplifies tasks like addition, subtraction, multiplication, and division using a simple syntax.
In this tutorial, we will explore how to use arithmetic expansion in Bash with practical examples.
Arithmetic expansion in Bash is done using the syntax
$((expression))
where expression
is the arithmetic operation you want to perform.
Basic Arithmetic Operations
Let’s start by demonstrating some basic arithmetic operations in Bash using arithmetic expansion.
Addition
To add two numbers in Bash, we use the +
operator within the arithmetic expansion syntax. Here’s an example of adding two numbers:
addition.sh
#!/bin/bash
# Define two numbers
num1=10
num2=5
# Add the numbers using arithmetic expansion
sum=$((num1 + num2))
# Output the result
echo "The sum of $num1 and $num2 is $sum"
Output
The sum of 10 and 5 is 15
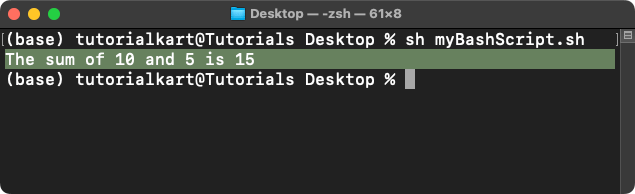
Subtraction
To subtract two numbers, use the -
operator in the same way:
subtraction.sh
#!/bin/bash
# Define two numbers
num1=10
num2=5
# Subtract the numbers using arithmetic expansion
difference=$((num1 - num2))
# Output the result
echo "The difference between $num1 and $num2 is $difference"
Output
The difference between 10 and 5 is 5
Multiplication
To multiply two numbers, use the *
operator with the arithmetic expansion.
multiplication.sh
#!/bin/bash
# Define two numbers
num1=10
num2=5
# Multiply the numbers using arithmetic expansion
product=$((num1 * num2))
# Output the result
echo "The product of $num1 and $num2 is $product"
Output
The product of 10 and 5 is 50
Division
To divide two numbers, use the /
operator. Note that in Bash, division involving integers will return an integer result (i.e., it will truncate the decimal part).
division.sh
#!/bin/bash
# Define two numbers
num1=10
num2=3
# Divide the numbers using arithmetic expansion
quotient=$((num1 / num2))
# Output the result
echo "The quotient of $num1 and $num2 is $quotient"
Output
The quotient of 10 and 3 is 3
Modulus (Remainder)
To find the remainder of a division operation, use the %
operator. This is known as the modulus operation:
modulus.sh
#!/bin/bash
# Define two numbers
num1=10
num2=3
# Modulus operation using arithmetic expansion
remainder=$((num1 % num2))
# Output the result
echo "The remainder when $num1 is divided by $num2 is $remainder"
Output
The remainder when 10 is divided by 3 is 1
Using Variables in Arithmetic Expansion
Arithmetic expansion in Bash can be used with variables as well. Simply assign values to variables and use them within the expansion. We have using variables in arithmetic expansion in all of the above examples.
Conclusion
In this tutorial, we’ve learned how to perform arithmetic operations in Bash using arithmetic expansion. We covered addition, subtraction, multiplication, division, and modulus, with examples demonstrating how to apply these operations to both literal numbers and variables. Arithmetic expansion is a powerful tool for basic calculations in Bash scripts, and it can help you automate tasks that involve numerical data.