Bash Average of Three Numbers using Arithmetic Expansion
In this tutorial, you will learn how to calculate the average of three numbers in Bash using arithmetic expansion. Arithmetic expansion is a simple and efficient way to perform calculations in Bash using the $(( expression ))
syntax.
The formula for calculating the average of three numbers is:
</>
Copy
average = (num1 + num2 + num3) / 3
We will demonstrate how to calculate the average by summing the three numbers and then dividing the result by 3, all using arithmetic expansion.
Average of Three Numbers Bash Program
Steps:
- Define three numbers: Three variables,
num1
,num2
, andnum3
, are initialized with the values10
,15
, and20
, respectively. - Calculate the average: The average of the three numbers is calculated using arithmetic expansion in Bash. The expression
((num1 + num2 + num3) / 3)
adds the three numbers together and divides the sum by 3 to compute the average. The result is assigned to the variableaverage
. - Output the result: The script uses the
echo
command to display the values of the three numbers and their calculated average on the console. Variable substitution ($num1
,$num2
,$num3
, and$average
) ensures the actual values are included in the output message.
Here’s a program that calculates the average of three numbers using arithmetic expansion.
Program
average_three.sh
</>
Copy
#!/bin/bash
# Define three numbers
num1=10
num2=15
num3=20
# Calculate the average using arithmetic expansion
average=$(( (num1 + num2 + num3) / 3 ))
# Output the result
echo "The average of $num1, $num2, and $num3 is $average"
Output
The average of 10, 15, and 20 is 15
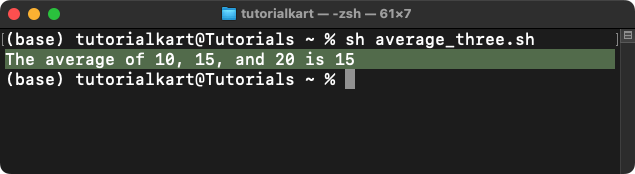