Average of Two Numbers in Bash
In this tutorial, you will learn how to calculate the average of two numbers in Bash using arithmetic expansion. We will also cover a program where we read the two numbers from user, and find their average.
The average of two given numbers is computed by summing the two numbers and then dividing the sum by 2.
Average of Two Numbers using Arithmetic Expansion
The simplest way to calculate the average of two numbers in Bash is to use arithmetic expansion. The formula for average is:
average = (num1 + num2) / 2
Here’s an example that calculates the average using arithmetic expansion.
average_arithmetic.sh
#!/bin/bash
# Define two numbers
num1=8
num2=12
# Calculate the average using arithmetic expansion
average=$(( (num1 + num2) / 2 ))
# Output the result
echo "The average of $num1 and $num2 is $average"
Output
The average of 8 and 12 is 10
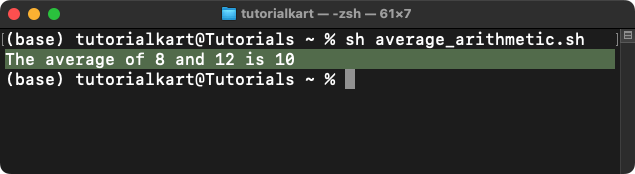
Example – Average of Two Numbers with User Input
We can also calculate the average by accepting user input for the two numbers. This allows for dynamic input and is more interactive. Here’s an example where the script asks the user to input the two numbers and then calculates their average.
average.sh
#!/bin/bash
# Prompt the user for two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Calculate the average using arithmetic expansion
average=$(( (num1 + num2) / 2 ))
# Output the result
echo "The average of $num1 and $num2 is $average"
Output
Enter the first number:
8
Enter the second number:
12
The average of 8 and 12 is 10
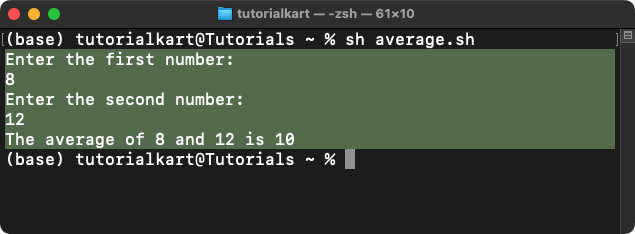