Bash – Check if Two Numbers are Equal
In Bash, you can use the -eq
operator to check if two numbers are equal.
Using -eq
to Check Equality of Two Numbers
The -eq
operator is used to compare two numbers. It can be used as a condition in if-else statement. If the numbers are equal, the condition evaluates to true, and the corresponding block of code is executed. If the numbers are not equal, then condition evaluates to false and the else block is executed.
Syntax
The syntax for the condition to check if num1
is equal to num2
is:
[ num1 -eq num2 ]
This condition can be used with conditional statements like if-else.
Bash Scripts
1 Checking if Two Predefined Numbers are Equal
This example demonstrates how to compare two predefined numbers in a Bash script using the -eq
operator.
Example
check_equal.sh
#!/bin/bash
num1=10
num2=10
if [ $num1 -eq $num2 ]; then
echo "The numbers are equal."
else
echo "The numbers are not equal."
fi
Output
The numbers are equal.
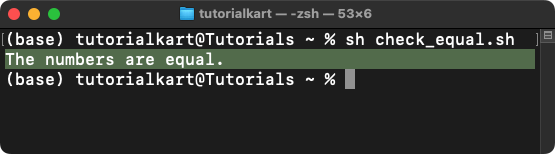
2 Accepting Numbers from User Input and Checking them for Equality
You can make the script dynamic by allowing users to input the numbers to compare. The script will then determine if the numbers are equal.
Example
check_equal.sh
#!/bin/bash
# Prompt the user to enter two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Compare the numbers
if [ $num1 -eq $num2 ]; then
echo "The numbers are equal."
else
echo "The numbers are not equal."
fi
Output
Enter the first number:
10
Enter the second number:
20
The numbers are not equal.
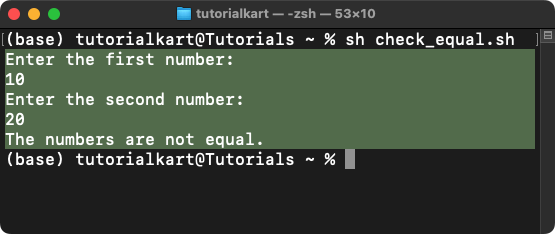
3 Nested Conditions – If Numbers are Equal, Check for Positive
This example demonstrates using -eq
in a script with nested conditions to perform additional checks based on the equality of two numbers.
Example
nested_check_equal.sh
#!/bin/bash
num1=10
num2=10
if [ $num1 -eq $num2 ]; then
echo "The numbers are equal."
if [ $num1 -gt 0 ]; then
echo "The numbers are also positive."
fi
else
echo "The numbers are not equal."
fi
Output
The numbers are equal.
The numbers are also positive.
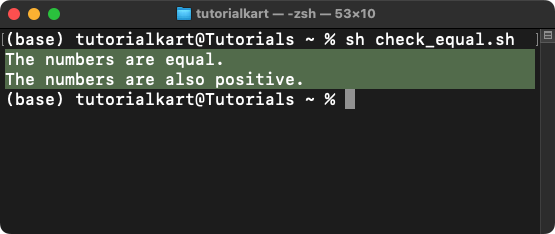