Check Prime Number in Bash
In this tutorial, you will learn how to check if a given number is a prime number in Bash.
A prime number is a number greater than 1 that has no divisors other than 1 and itself.
The basic logic to check if a number is prime involves checking if the number is divisible by any number other than 1 and itself. If it is divisible, the number is not prime. If it is not divisible by any other number, it is a prime number.
Program
check_prime.sh
</>
Copy
#!/bin/bash
# Prompt the user for a number
echo "Enter a number:"
read num
# Check if the number is less than or equal to 1
if [ $num -le 1 ]; then
echo "$num is not a prime number."
exit
fi
# Check divisibility from 2 to the square root of the number
for (( i=2; i<=$((num / 2)); i++ ))
do
if [ $((num % i)) -eq 0 ]; then
echo "$num is not a prime number."
exit
fi
done
# If no divisor is found, it's a prime number
echo "$num is a prime number."
Steps Explanation:
- Prompt the user for a number: The script displays the message
"Enter a number:"
using theecho
command and reads the input into the variablenum
using theread
command. - Handle edge cases: The script first checks if the number is less than or equal to 1. If it is, the script displays
"Not a prime number"
as prime numbers are greater than 1. - Check divisibility: The script checks for divisibility from 2 up to the square root of the number (to reduce unnecessary checks). If the number is divisible by any of these, it is not a prime number.
- Return result: Based on the divisibility checks, the script prints whether the number is prime or not.
Output:
Enter a number:
7
7 is a prime number.
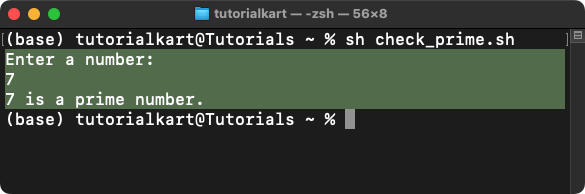
Enter a number:
10
10 is not a prime number.
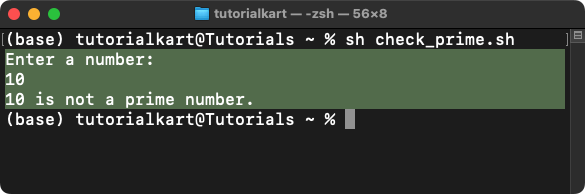