Bash -eq
Operator
The -eq
operator in Bash is used to check if two numbers are equal.
This operator is part of the comparison operators provided by Bash for performing numeric comparisons.
Syntax of Equal Operator
[ num1 -eq num2 ]
Here, num1
and num2
are the numbers being compared. The condition returns true if the two numbers are equal.
If the numbers are equal, the condition evaluates to true; otherwise, it evaluates to false.
The -eq
operator is commonly used in conditional statements like if
and while
.
Examples of -eq
(Equal To)Operator
Example 1: Checking Equality Between Two Predefined Numbers
In this example, we take two predefined in two variables, or say hard coded numbers, and check they are equal.
Program
check_equal.sh
#!/bin/bash
# Define two numbers
num1=10
num2=10
# Compare the numbers
if [ $num1 -eq $num2 ]; then
echo "The numbers are equal."
else
echo "The numbers are not equal."
fi
Steps:
- Define two numbers: The script initializes two variables,
num1
andnum2
, with the value10
each. - Compare the numbers: The
if
statement uses the-eq
operator to check if the values ofnum1
andnum2
are equal. - Execute the true condition: If the condition evaluates to true (the numbers are equal), the script executes the
echo
command to display the message"The numbers are equal."
. - Execute the false condition: If the condition evaluates to false (the numbers are not equal), the
else
block executes, and the script displays the message"The numbers are not equal."
.
Output
The numbers are equal.
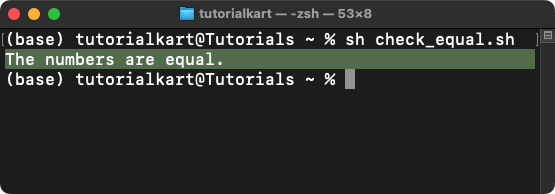
Example 2: Accepting User Input and Comparing those Two Numbers
In this example, we shall read the two numbers from user, and compare them if they are equal.
Program
check_equal.sh
#!/bin/bash
# Prompt the user to enter two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Compare the numbers
if [ $num1 -eq $num2 ]; then
echo "The numbers are equal."
else
echo "The numbers are not equal."
fi
Steps:
- Prompt the user to enter the first number: The script displays the message
"Enter the first number:"
using theecho
command and reads the input into the variablenum1
using theread
command. - Prompt the user to enter the second number: Similarly, the script prompts the user with the message
"Enter the second number:"
and reads the input into the variablenum2
. - Once we have the two numbers, comparing them using an if-else is same as that of in the previous program.
Output
Enter the first number:
10
Enter the second number:
20
The numbers are not equal.
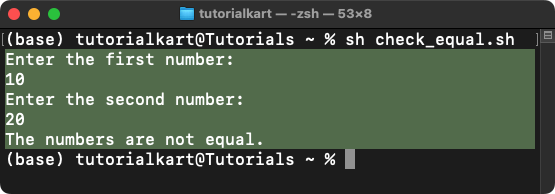
Example 3: Using the -eq
Operator in a Loop
In this example we shall iterate over a range of numbers from 1 to 10, and check if the number in the iteration is equal to that of the given target.
Program
check_equal.sh
#!/bin/bash
# Define the target number
target=5
# Loop from 1 to 10
for num in {1..10}; do
if [ $num -eq $target ]; then
echo "$num is equal to the target number."
else
echo "$num is not equal to the target number."
fi
done
Steps:
- Define the target number: The script initializes a variable
target
with the value5
. - Start a loop from 1 to 10: The
for
loop iterates over the numbers from1
to10
, assigning each number to the variablenum
. - Compare each number with the target: Inside the loop, the
if
statement uses the-eq
operator to check if the current value ofnum
is equal to the value oftarget
. - Execute the true condition: If
num
equalstarget
, the script executes theecho
command to display the message"$num is equal to the target number."
. - Execute the false condition: If
num
does not equaltarget
, theelse
block executes, and the script displays the message"$num is not equal to the target number."
. - Repeat for all numbers: Steps 3–5 are repeated for every number in the range from
1
to10
.
Output
1 is not equal to the target number.
2 is not equal to the target number.
3 is not equal to the target number.
4 is not equal to the target number.
5 is equal to the target number.
6 is not equal to the target number.
7 is not equal to the target number.
8 is not equal to the target number.
9 is not equal to the target number.
10 is not equal to the target number.
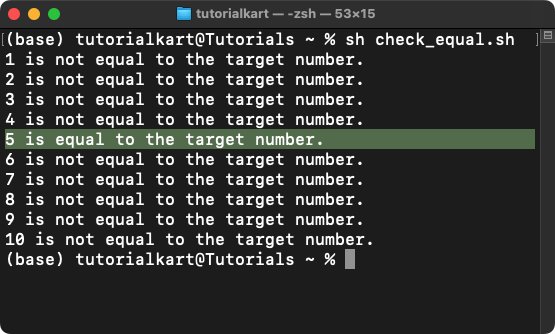