Bash -gt
Operator
The -gt
operator in Bash is used to check if one number is greater than another. The operator takes two operands, and checks if the first number is greater than the second number.
This operator is part of the comparison operators provided by Bash for performing numeric comparisons.
Syntax of -gt
Operator
The syntax of -gt
operator with operands is:
[ num1 -gt num2 ]
Here, num1
and num2
are the numbers being compared. The condition returns true if num1
is greater than num2
.
If the first number is greater, the condition evaluates to true; otherwise, it evaluates to false.
The -gt
operator is commonly used in conditional statements like if
and while
.
Examples of -gt
(Greater Than) Operator
Example 1: Checking if a Number is Greater Than Another
In this example, we take two predefined numbers and check if the first number is greater than the second number using the -gt
operator.
Program
check_greater.sh
#!/bin/bash
# Define two numbers
num1=15
num2=10
# Compare the numbers
if [ $num1 -gt $num2 ]; then
echo "The first number is greater."
else
echo "The first number is not greater."
fi
Steps:
- Define two numbers: The script initializes two variables,
num1
andnum2
, with values15
and10
, respectively. - Compare the numbers: The
if
statement uses the-gt
operator to check if the value ofnum1
is greater thannum2
. - Execute the true condition: If the condition evaluates to true (the first number is greater), the script executes the
echo
command to display the message"The first number is greater."
. - Execute the false condition: If the condition evaluates to false (the first number is not greater), the
else
block executes, and the script displays the message"The first number is not greater."
.
Output
The first number is greater.
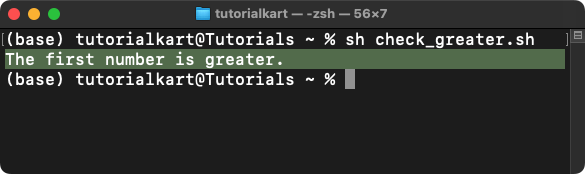
Example 2: Accepting User Input and Comparing Two Numbers
In this example, we will read two numbers from the user and compare them to check if the first number is greater than the second.
Program
check_greater.sh
#!/bin/bash
# Prompt the user to enter two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Compare the numbers
if [ $num1 -gt $num2 ]; then
echo "The first number is greater."
else
echo "The first number is not greater."
fi
Steps:
- Prompt the user to enter the first number: The script displays the message
"Enter the first number:"
using theecho
command and reads the input into the variablenum1
using theread
command. - Prompt the user to enter the second number: Similarly, the script prompts the user with the message
"Enter the second number:"
and reads the input into the variablenum2
. - Once we have the two numbers, we compare them using the
-gt
operator to check if the first number is greater than the second.
Output
Enter the first number:
20
Enter the second number:
10
The first number is greater.
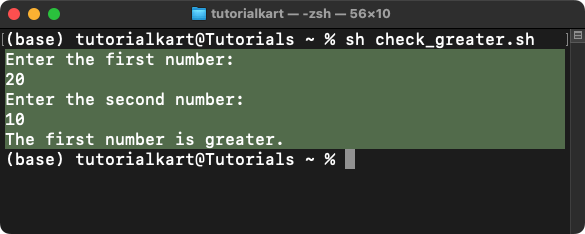
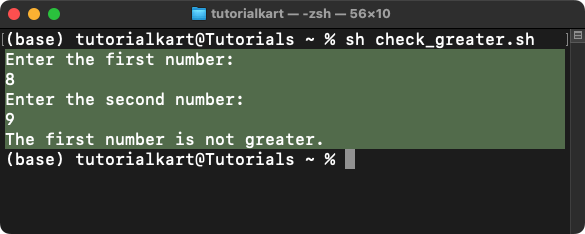