Find Largest of Three Numbers in Bash
In this tutorial, you will learn how to find the largest of three numbers in a Bash script using nested if
statements or a combination of if
, elif
, and else
, along with comparison operators.
Using If-Elif-Else Statement to Find the Largest of Three Numbers
We can use the if
statement along with elif
and else
to compare three numbers. The logic involves checking the relationship between the numbers step by step:
- If the first number is greater than or equal to the second and third numbers, it is the largest.
- If the second number is greater than or equal to third number, it is the largest.
- Otherwise, the third number is the largest.
The comparison is done using the -ge
operator, which stands for “greater than or equal to”.
Here’s an example of how to implement this logic in Bash:
largest_of_three.sh
</>
Copy
#!/bin/bash
# Define three numbers
num1=10
num2=20
num3=15
# Compare the three numbers to find the largest
if [ $num1 -ge $num2 ] && [ $num1 -ge $num3 ]; then
echo "$num1 is the largest number."
elif [ $num2 -ge $num3 ]; then
echo "$num2 is the largest number."
else
echo "$num3 is the largest number."
fi
Explanation:
- Define three numbers: Three variables,
num1
,num2
, andnum3
, are initialized with the values10
,20
, and15
, respectively. - Check the conditions:
- The first
if
condition checks ifnum1
is greater than or equal to bothnum2
andnum3
. If true,num1
is the largest. - The
elif
condition checks ifnum2
is greater than or equal tonum3
. If true,num2
is the largest. - If both conditions are false, the
else
block executes, indicating thatnum3
is the largest.
- The first
- Output the largest number: The
echo
command is used to print the largest number to the console. - End of script: The script ends after determining and displaying the largest number.
Output
20 is the largest number.
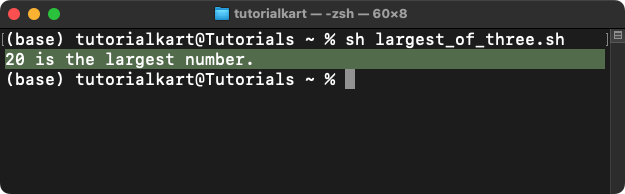
To make the script more dynamic, let’s modify it to accept user input for the three numbers. This allows the user to enter any three numbers and determine the largest.
largest_of_three.sh
</>
Copy
#!/bin/bash
# Prompt the user to enter three numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
echo "Enter the third number:"
read num3
# Compare the three numbers to find the largest
if [ $num1 -ge $num2 ] && [ $num1 -ge $num3 ]; then
echo "$num1 is the largest number."
elif [ $num2 -ge $num1 ] && [ $num2 -ge $num3 ]; then
echo "$num2 is the largest number."
else
echo "$num3 is the largest number."
fi
Explanation:
- Prompt the user for input:
- The script prompts the user to enter the first number and reads it into
num1
. - The user is then prompted to enter the second and third numbers, which are read into
num2
andnum3
, respectively.
- The script prompts the user to enter the first number and reads it into
- The rest of the steps are the same as in the previous script.
Example Output:
Enter the first number:
25
Enter the second number:
10
Enter the third number:
15
25 is the largest number.
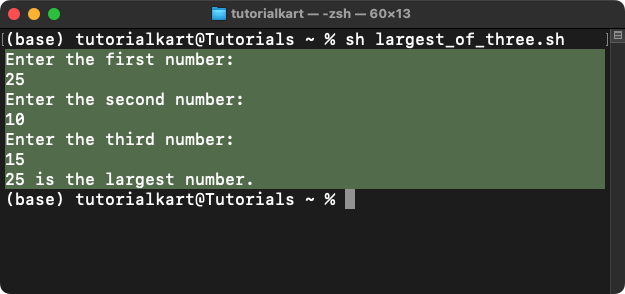