Logical Operators in Bash
In this tutorial, you will learn about logical operators in Bash. Logical operators allow you to perform conditional logic by combining multiple conditions. They are commonly used in if
, elif
, and while
statements to make decisions in your Bash scripts.
Types of Logical Operators in Bash
Bash supports three main types of logical operators:
- AND Operator (
&&
or-a
): Evaluates to true if both conditions are true. - OR Operator (
||
or-o
): Evaluates to true if at least one condition is true. - NOT Operator (
!
): Reverses the result of a condition.
Using Logical Operators in Bash
Logical operators are often used in if
statements to perform compound conditional checks. Let’s explore each operator with examples.
AND Operator (&&
)
The AND operator returns true only if both conditions are true. In Bash, you can use &&
or -a
to represent the AND operator.
Example: Check if a number is between 10 and 20:
example.sh
#!/bin/bash
num=15
if [ $num -ge 10 ] && [ $num -le 20 ]; then
echo "The number $num is between 10 and 20."
else
echo "The number $num is not between 10 and 20."
fi
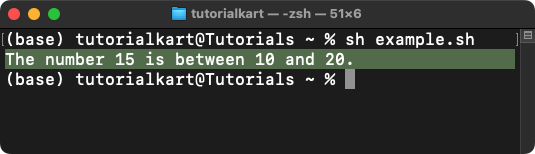
OR Operator (||
)
The OR operator returns true if at least one of the conditions is true. In Bash, you can use ||
or -o
to represent the OR operator.
Example: Check if a number is less than 10 or greater than 20:
example.sh
#!/bin/bash
num=25
if [ $num -lt 10 ] || [ $num -gt 20 ]; then
echo "The number $num is either less than 10 or greater than 20."
else
echo "The number $num is between 10 and 20."
fi
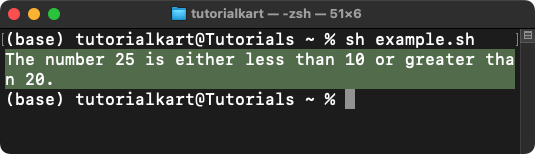
NOT Operator (!
)
The NOT operator reverses the result of a condition. It returns true if the condition is false.
Example: Check if a directory does not exist:
example.sh
#!/bin/bash
dir="my_directory"
if [ ! -d $dir ]; then
echo "The directory $dir does not exist."
else
echo "The directory $dir exists."
fi
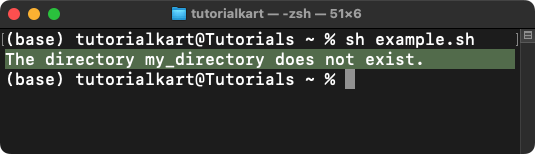
Combining Logical Operators
You can combine multiple logical operators to create complex conditions. Parentheses ()
can be used to group conditions and control the order of evaluation.
Example: Check if a number is between 10 and 20 or greater than 30:
example.sh
#!/bin/bash
num=35
if ([ $num -ge 10 ] && [ $num -le 20 ]) || [ $num -gt 30 ]; then
echo "The number $num is either between 10 and 20 or greater than 30."
else
echo "The number $num does not satisfy the conditions."
fi
Explanation:
- AND operator: The first condition checks if the number is between 10 and 20 using
&&
. - OR operator: The second condition checks if the number is greater than 30 using
||
. - Combination: The overall condition combines these checks, ensuring flexibility in evaluating the number.