Bash -ne
Operator
The -ne
operator in Bash is used to check if two numbers are “not equal” to each other.
This operator is part of the comparison operators provided by Bash for performing numeric comparisons.
Syntax of -ne
Operator
The syntax of -ne
operator with operands is:
</>
Copy
[ num1 -ne num2 ]
Here, num1
and num2
are the numbers being compared. The condition returns true if the two numbers are not equal.
If the numbers are not equal, the condition evaluates to true; otherwise, it evaluates to false.
The -ne
operator is commonly used in conditional statements like if
and while
.
Examples of -ne
(Not Equal To) Operator
Example 1: Checking If Two Predefined Numbers Are Not Equal
In this example, we take two predefined numbers in variables and check if they are not equal.
Program
check_unequal.sh
</>
Copy
#!/bin/bash
# Define two numbers
num1=10
num2=20
# Compare the numbers
if [ $num1 -ne $num2 ]; then
echo "The numbers are not equal."
else
echo "The numbers are equal."
fi
Steps:
- Define two numbers: The script initializes two variables,
num1
andnum2
, with values10
and20
, respectively. - Compare the numbers: The
if
statement uses the-ne
operator to check if the values ofnum1
andnum2
are not equal. - Execute the true condition: If the condition evaluates to true (the numbers are not equal), the script executes the
echo
command to display the message"The numbers are not equal."
. - Execute the false condition: If the condition evaluates to false (the numbers are equal), the
else
block executes, and the script displays the message"The numbers are equal."
. - End of script: After determining the inequality and printing the appropriate message, the script terminates.
Output
The numbers are not equal.
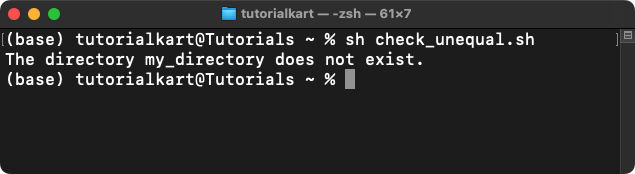
Example 2: Accepting User Input and Comparing Two Numbers
In this example, we will read two numbers from the user and compare them to check if they are not equal.
Program
check_unequal.sh
</>
Copy
#!/bin/bash
# Prompt the user to enter two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Compare the numbers
if [ $num1 -ne $num2 ]; then
echo "The numbers are not equal."
else
echo "The numbers are equal."
fi
Steps:
- Prompt the user to enter the first number: The script displays the message
"Enter the first number:"
using theecho
command and reads the input into the variablenum1
using theread
command. - Prompt the user to enter the second number: Similarly, the script prompts the user with the message
"Enter the second number:"
and reads the input into the variablenum2
. - Once we have the two numbers, we compare them using the
-ne
operator to check if they are not equal.
Output
Enter the first number:
10
Enter the second number:
20
The numbers are not equal.
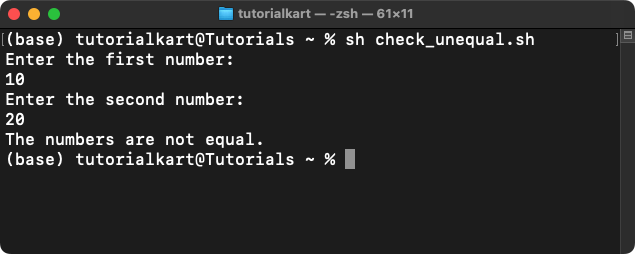
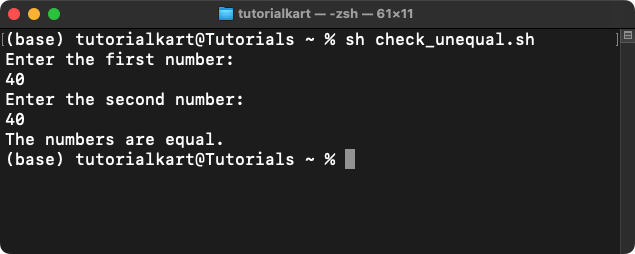