Bash !
(NOT) Operator
The !
operator in Bash is used to negate a condition. It is commonly referred to as the “NOT” operator.
This operator inverts the exit status of a command or a conditional expression. In simple terms, if a command or expression returns true (exit status 0), the !
operator changes it to false (non-zero exit status), and if it returns false (non-zero exit status), it changes it to true (exit status 0).
The !
operator is commonly used in conditional statements to check the opposite of a condition or to negate a condition. It can be used with if
statements, loops, and other conditional expressions.
Syntax of NOT Operator
if ! command; then
# commands to run if the command fails
fi
Here, the !
is placed before a command or condition. If the command or condition returns a failure (non-zero exit status), the !
negates it and makes the if
condition true.
The !
operator is typically used with commands or test expressions to perform a logical negation. If the command returns false, the negation makes it true.
The !
operator can be used in various places where Bash expects a condition, such as within if
statements, while
loops, and with the test
command.
Examples of !
(NOT) Operator
Example 1: Using !
to Negate a Command
In this example, we will use the !
operator to negate the success of a command. If the command fails (non-zero exit status), we can use !
to perform an action when the command fails.
Program
check_directory.sh
#!/bin/bash
# Try to create a directory
mkdir /tmp/test_directory
# Check if the command fails using the NOT operator
if ! mkdir /tmp/test_directory; then
echo "Directory could not be created, it may already exist."
else
echo "Directory created successfully."
fi
Steps Explanation:
- The script uses the
!
operator to negate the success of themkdir
command. - If the
mkdir
command fails (for example, if the directory already exists), theif
condition will be true and the script will output that the directory could not be created.
Output
Directory could not be created, it may already exist.
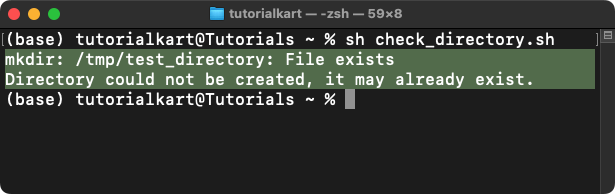
Example 2: Using !
with test
Command
The test
command can be used to evaluate conditions in Bash scripts. We can use the !
operator with test
to negate conditions. In this example, we will check if a file exists using the test
command and negate the result to check if the file does not exist.
Program
check_file.sh
#!/bin/bash
# Check if the file does not exist
if ! test -f /tmp/test_file.txt; then
echo "File does not exist."
else
echo "File exists."
fi
Steps Explanation:
- The script checks if the file
/tmp/test_file.txt
does not exist using the!
operator withtest
. - If the file does not exist, the script will output that the file is missing.
Output
File does not exist.
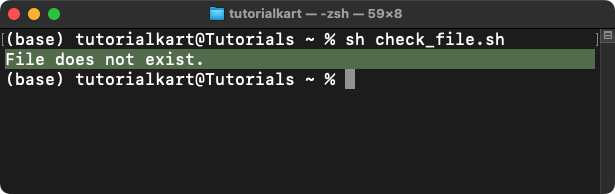