Print Numbers from 1 to N in Bash Scripting
In this tutorial, you will learn how to print numbers from 1 to N in a Bash script using loops, specifically the for
loop in Bash.
Using a For Loop to Print Numbers from 1 to N
The most common way to print numbers from 1 to N is by using a for loop.
Here’s an example that prints numbers from 1 to N:
print_numbers.sh
</>
Copy
#!/bin/bash
# Prompt the user for the value of N
echo "Enter the number N:"
read N
# Print numbers from 1 to N using a for loop
for ((i=1; i<=N; i++))
do
echo $i
done
Output
Enter the number N:
5
1
2
3
4
5
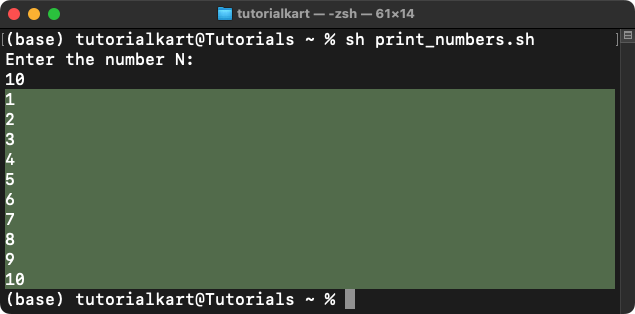
Explanation:
- Prompt the user for input: The script uses the
echo
command to display the message"Enter the number N:"
, prompting the user to input a value forN
. - Read the user input: The
read
command captures the user’s input and stores it in the variableN
. - Use a
for
loop with C-style syntax: Thefor
loop is defined using C-style syntax:- Initialization:
i=1
, starting the loop from 1. - Condition:
i<=N
, ensuring the loop continues as long asi
is less than or equal toN
. - Increment:
i++
, incrementing the value ofi
by 1 in each iteration.
- Initialization:
- Print each number: During each iteration of the loop, the current value of
i
is printed to the console using theecho
command. - End of script: Once the loop completes (after printing all numbers from
1
toN
), the script ends successfully.
Using a For Loop with a Range to Print Numbers from 1 to N
Alternatively, you can use a range in the for
loop to print numbers from 1 to N. This method uses the {}
syntax in the loop declaration:
</>
Copy
for i in {1..N}
Here’s an example of how to print numbers from 1 to N using this method:
print_numbers.sh
</>
Copy
#!/bin/bash
# Prompt the user for the value of N
echo "Enter the number N:"
read N
# Print numbers from 1 to N using a range in the for loop
for i in $(seq 1 $N)
do
echo $i
done
Output
Enter the number N:
5
1
2
3
4
5
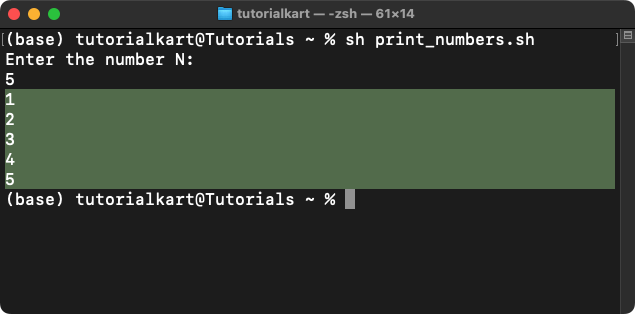
Explanation:
- Prompt the user for input: The script displays the message
"Enter the number N:"
to prompt the user to input a value forN
. - Read the user input: The
read
command takes the user’s input and assigns it to the variableN
. - Use a
for
loop to iterate from 1 toN
: Theseq
command generates a sequence of numbers from1
toN
. Thefor
loop iterates over this sequence, assigning each value to the variablei
. - Print each number: During each iteration, the script uses the
echo
command to print the current value ofi
to the console. - End of script: Once the loop completes (after printing all numbers from
1
toN
), the script ends successfully.