Find Smallest of Two Numbers in Bash
In this tutorial, you will learn how to find the smallest of two numbers in a Bash script using if-else conditional statement with the comparison operators.
Using If-Else Statement to Find the Smallest of Two Numbers
The simplest way to find the smallest of two numbers in Bash is by using an if-else
statement to check if the first number is less than or equal to the second number. If the condition is true, we know that the first number is the smallest. Otherwise, the second number is the smallest.
The -le
operator is used in Bash to compare two numbers. The -le
operator stands for “less than or equal to” and checks whether the first number is less than or equal to the second number.
Here’s an example that uses the -le
operator to find the smallest of two numbers in an if-else statement:
smallest_number.sh
#!/bin/bash
# Define two numbers
num1=10
num2=5
# Compare the two numbers and find the smallest
if [ $num1 -le $num2 ]; then
echo "$num1 is the smallest number."
else
echo "$num2 is the smallest number."
fi
Explanation:
- Defining two numbers: Two variables,
num1
andnum2
, are initialized with the values10
and5
, respectively. - Comparing the two numbers: The
if
condition uses the-le
(less than or equal to) operator to comparenum1
andnum2
:- If
num1
is less than or equal tonum2
, the condition evaluates to true. - Otherwise, the condition evaluates to false, and the script executes the
else
block.
- If
- Printing the smallest number:
- If the condition is true, the script prints
"$num1 is the smallest number."
using theecho
command. - If the condition is false, the script prints
"$num2 is the smallest number."
.
- If the condition is true, the script prints
- End of script: The script ends after successfully determining and displaying the smallest number.
Output
5 is the smallest number.
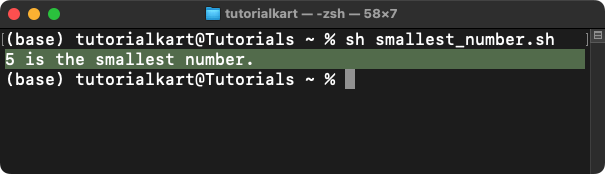
Now, let’s modify the script to accept user input for the two numbers. This makes the script more dynamic and allows the user to enter any pair of numbers to find the smallest.
smallest_number.sh
#!/bin/bash
# Prompt the user to enter two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Compare the two numbers and find the smallest
if [ $num1 -le $num2 ]; then
echo "$num1 is the smallest number."
else
echo "$num2 is the smallest number."
fi
Explanation:
- Prompt the user for input:
- The script prompts the user to enter the first number using
echo "Enter the first number:"
and reads the input into the variablenum1
using theread
command. - It then prompts the user to enter the second number using
echo "Enter the second number:"
and reads the input into the variablenum2
.
- The script prompts the user to enter the first number using
- Rest of the steps are the same as in the previous program.
Example Output:
Enter the first number:
8
Enter the second number:
15
8 is the smallest number.
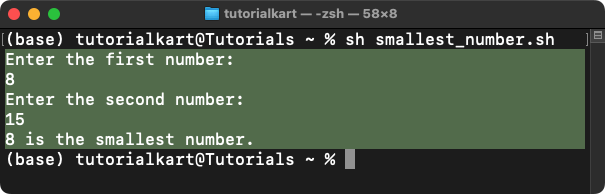