Sum of Three Numbers in Bash
You can find the sum of three numbers in bash using arithmetic expansion, or the let
command.
In this tutorial, you will learn how to find the sum of three numbers in Bash using various approaches. We will demonstrate how to do this using arithmetic expansion, the let
command, and reading user input.
Approach 1: Sum of Three Numbers using Arithmetic Expansion
We will start by using arithmetic expansion to sum three numbers. Arithmetic expansion is a simple way to perform calculations in Bash using the $((expression))
syntax.
sum_arithmetic.sh
#!/bin/bash
# Define three numbers
num1=5
num2=3
num3=7
# Sum the numbers using arithmetic expansion
sum=$((num1 + num2 + num3))
# Output the result
echo "The sum of $num1, $num2, and $num3 is $sum"
Output
The sum of 5, 3, and 7 is 15
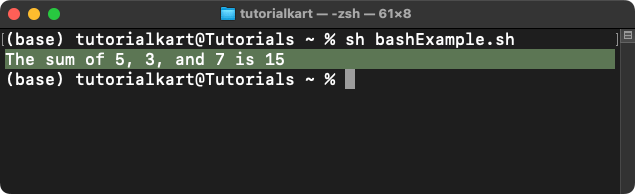
Approach 2: Sum of Three Numbers using let Command
Next, we will use the let
command to sum three numbers. The let
command allows you to perform arithmetic operations directly on variables without using the $(( ))
syntax.
sum_let.sh
#!/bin/bash
# Define three numbers
num1=5
num2=3
num3=7
# Sum the numbers using let command
let sum=num1+num2+num3
# Output the result
echo "The sum of $num1, $num2, and $num3 is $sum"
Output
The sum of 5, 3, and 7 is 15
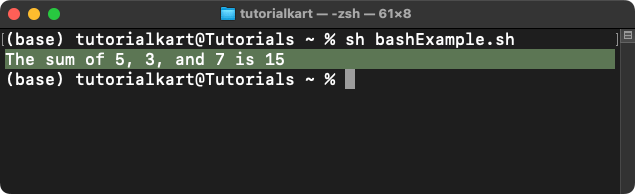
Example – Sum of Three Numbers with User Input
Finally, we will create a script that asks the user to input three numbers, then sums them.
sum_input.sh
#!/bin/bash
# Prompt the user for three numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
echo "Enter the third number:"
read num3
# Sum the numbers using arithmetic expansion
sum=$((num1 + num2 + num3))
# Output the result
echo "The sum of $num1, $num2, and $num3 is $sum"
Output
Enter the first number:
5
Enter the second number:
3
Enter the third number:
7
The sum of 5, 3, and 7 is 15
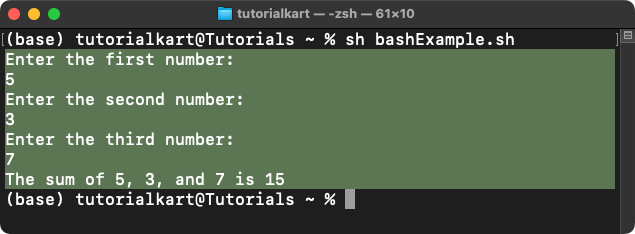