Sum of Two Numbers Program in Bash
In Bash, adding two numbers can be easily done using arithmetic operations. This tutorial will guide you through the steps to write a program that sums two numbers in Bash.
We will demonstrate the process using different methods for summing numbers, along with practical examples.
Sum of Two Numbers using Arithmetic Expansion
One of the simplest ways to perform arithmetic in Bash is by using arithmetic expansion. The syntax for arithmetic expansion is
</>
Copy
$((expression))
And the expression to find the sum of two numbers is
</>
Copy
$((num1 + num2))
sum.sh
</>
Copy
#!/bin/bash
# Define two numbers
num1=5
num2=3
# Sum the numbers using arithmetic expansion
sum=$((num1 + num2))
# Output the result
echo "The sum of $num1 and $num2 is $sum"
Output
The sum of 5 and 3 is 8
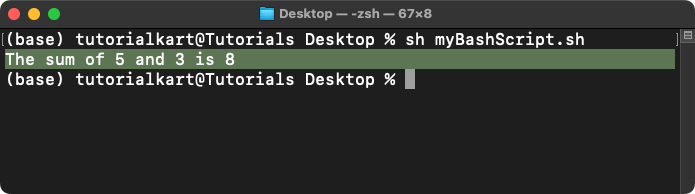
Sum of Two Numbers using let Command
The let
command in Bash can be used for arithmetic operations as well. This method allows you to directly modify variables without needing the $(( ))
syntax.
sum.sh
</>
Copy
#!/bin/bash
# Define two numbers
num1=5
num2=3
# Sum the numbers using let command
let sum=num1+num2
# Output the result
echo "The sum of $num1 and $num2 is $sum"
Output
The sum of 5 and 3 is 8
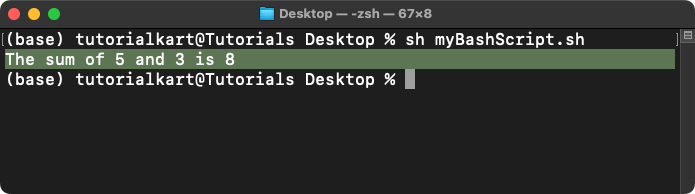