In this C++ tutorial, you will learn how to check if a given string contains a specific substring value, with example programs.
Check if the string contains a specific substring in C++
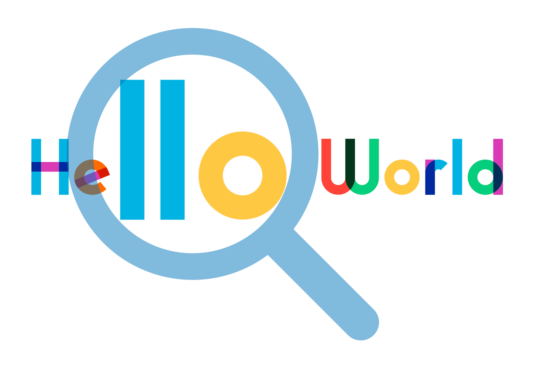
To check if the given string contains a specific substring in C++, you can use find() method of string class.
1. Checking if string contains substring using find() method in C++
In the following program, we are given a string in str and the substring in substring. We have to check if the string str contains substring using find() method.
Steps
- Given an input string in
str
, and a specific substring insubstring
. - Call the
find()
method of thestring
class to search for thesubstring
within thestr
. - If the
find()
method returns a value other thanstring::npos
, it means the substring was found, indicating thatstr
contains thesubstring
. Use this information to create a condition that returns true only if the stringstr
contains thesubstring
. - Write a C++ if else statement with the condition created from the previous step.
Program
main.cpp
</>
Copy
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World";
string substring = "llo";
if (str.find(substring) != string::npos) {
cout << "The string contains the substring." << endl;
} else {
cout << "The string does not contain the substring." << endl;
}
return 0;
}
Output
The string contains the substring.
Now, let us change the substring value such that the string str does not contain substring, and run the program again.
main.cpp
</>
Copy
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello World";
string substring = "apple";
if (str.find(substring) != string::npos) {
cout << "The string contains the substring." << endl;
} else {
cout << "The string does not contain the substring." << endl;
}
return 0;
}
Output
The string does not contain the substring.
Conclusion
In this C++ Tutorial, we learned how to check if the string contains the specified substring value using string find() method, with examples.