C++ Get Current Time using ctime Library
In this C++ tutorial, you will learn how to get the current time using the ctime library, with examples and detailed explanations.
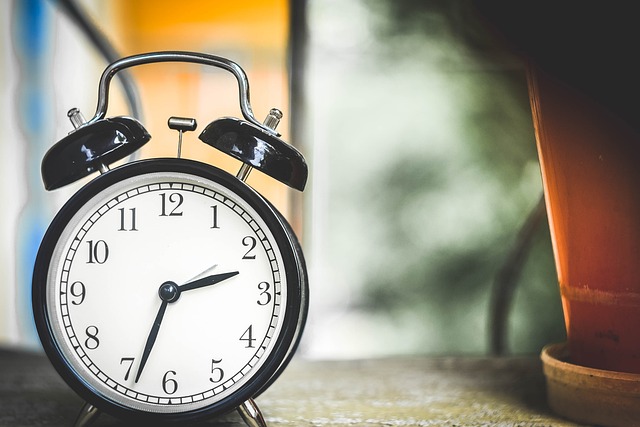
C++ ctime Library
The ctime header file in C++ provides various functions to handle date and time. One of its most commonly used functions is time(), which retrieves the current system time.
The time()
function returns the time in seconds since the epoch (January 1, 1970). To format this into a human-readable string, the ctime()
function is used, which converts the time value into a string representation.
To use these time-related functions in your program, include the ctime header file. You may also need iostream for input/output operations and std namespace for cleaner syntax.
Example: Getting Current Time in C++
In the following example, we will retrieve and display the current time using the time() and ctime() functions from the ctime library.
C++ Program
#include <iostream>
#include <ctime>
using namespace std;
int main() {
// Get current time as time_t object
time_t now = time(0);
// Convert to human-readable string
char* dt = ctime(&now);
cout << "The current local time is: " << dt << endl;
return 0;
}
Output
The current local time is: Wed Nov 15 11:30:45 2024
Explanation of the Code
Here’s a breakdown of the code:
1. #include <ctime>
: This header file is used to handle date and time operations. It provides functions like time()
and ctime()
.
2. time_t now = time(0);
: The time()
function retrieves the current time in seconds since the epoch. The return value is stored in a time_t
object, which is a data type for time values.
3. char* dt = ctime(&now);
: The ctime()
function converts the time value into a human-readable string format. It returns a C-style string containing the current time and date.
4. cout
: This is used to print the formatted time to the console.
Formatting Time for Custom Output
You can customize the way time is displayed by using the tm structure and the strftime() function for formatted output. Here’s an example:
C++ Program
#include <iostream>
#include <ctime>
using namespace std;
int main() {
// Get current time
time_t now = time(0);
// Convert to struct tm
tm* localTime = localtime(&now);
cout << "Year: " << 1900 + localTime->tm_year << endl;
cout << "Month: " << 1 + localTime->tm_mon << endl;
cout << "Day: " << localTime->tm_mday << endl;
cout << "Time: " << localTime->tm_hour << ":"
<< localTime->tm_min << ":"
<< localTime->tm_sec << endl;
return 0;
}
Output
Year: 2024
Month: 11
Day: 15
Time: 11:30:45
Key Functions in ctime
time(): Returns the current time as a time_t
object.
ctime(): Converts a time_t
object into a human-readable string format.
localtime(): Converts a time_t
object into a tm
structure representing local time.
strftime(): Formats the time data into a custom string representation.
By using these functions, you can manipulate and display time in various formats according to your needs.