In this C++ tutorial, you will learn how to get the first N characters in a given string, with example programs.
Get first N characters in String in C++
To get the first N characters from a given string in C++, you can use substr() method of string class.
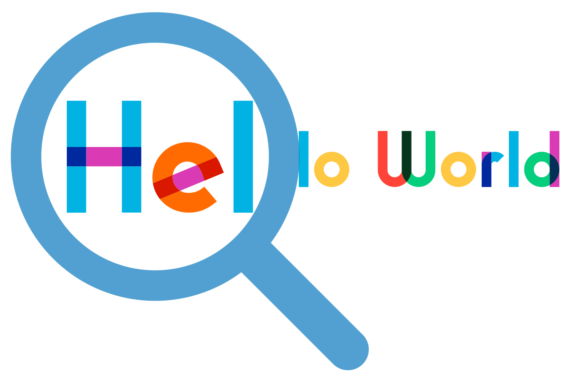
1. Getting the first N characters in string using substr() method in C++
In the following program, we are given a string in str and an integer value in n. We have to get the first n characters from the string str using substr() method.
Steps
- Given an input string in
str
, and an integer value inn
. - Call the
substr()
method on the stringstr
, and pass0
for the first parameter, andn
for the second parameter. - The
substr()
method returns the substring in the string from the index position of0
up to the index position ofn
. - Store the returned value in a variable, say
firstNChars
, and use it as per your requirement.
Program
main.cpp
</>
Copy
#include <iostream>
#include <string>
using namespace std;
int main() {
// Given string
string str = "Hello World!";
// Number of first characters to extract
int n = 3;
// Use substr to get the first n characters
string firstNChars = str.substr(0, n);
cout << "First " << n << " characters: " << firstNChars << endl;
return 0;
}
Output
First 3 characters: Hel
Now, let us try with another value of n
, say 7, and get the first n characters in the string.
main.cpp
</>
Copy
#include <iostream>
#include <string>
using namespace std;
int main() {
// Given string
string str = "Hello World!";
// Number of first characters to extract
int n = 7;
// Use substr to get the first n characters
string firstNChars = str.substr(0, n);
cout << "First " << n << " characters: " << firstNChars << endl;
return 0;
}
Output
First 7 characters: Hello W
Conclusion
In this C++ Tutorial, we learned how to get the first n characters in a string using string substr() method, with examples.