EXPERIMENT-3: HTML 5 and Cascading Style Sheets, Types of CSS
a. Write an HTML program that makes use of<article>
, <aside>
, <figure>
,<figcaption>
<footer>
, <header>
,<main>
, <nav>
, <section>
, <div>
, <span>
tags.
b. Write an HTML program to embed audio and video into an HTML web page.
c. Write a program to apply different types (or levels of styles or style specification formats) — inline, internal, external styles to HTML elements. (Identify selector, property, and value.)
a. Write an HTML program that makes use of
<article>, <aside>, <figure>, <figcaption>,
<footer>, <header>, <main>, <nav>, <section>,
<div>, <span> tags.
PROCEDURE STEPS:
Step 1: Open the Visual Studio Code and create a HTML file named index.html file. Write HTML content using the:
<article>, <aside>,
<figure>, <figcaption>,
<footer>, <header>,
<main>, <nav>,
<section>, <div>,
<span> tags.
Step 2: Locate the HTML file in File Explorer.
Step 3: Open the HTML in a Web Browser.
SOURCE CODE
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>HTML5 Tag Demonstration</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
header {
background-color: #007BFF;
color: white;
padding: 1rem;
text-align: center;
}
nav {
background-color: #f4f4f4;
padding: 1rem;
text-align: center;
}
nav a {
margin: 0 10px;
text-decoration: none;
color: #333;
}
main {
margin: 1rem;
}
div.row {
display: flex;
}
article, aside {
border: 1px solid #ddd;
padding: 1rem;
margin: 1rem;
flex: 2;
min-width: 300px;
}
aside {
flex: 1;
}
figure {
margin: 0;
text-align: center;
width: -webkit-fill-available;
}
figcaption {
font-size: 0.9rem;
color: #555;
}
img {
width: -webkit-fill-available;
}
footer {
background-color: #333;
color: white;
text-align: center;
padding: 1rem;
margin-top: 1rem;
}
</style>
</head>
<body>
<header>
<h1>Welcome to My HTML5 Demo Page</h1>
</header>
<nav>
<a href="#home">Home</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
<main>
<section>
<h2>About This Page</h2>
<p>This page demonstrates the use of various HTML5 tags such as <span style="color:blue;">header</span>, <span style="color:blue;">article</span>, <span style="color:blue;">figure</span>, and others.</p>
</section>
<div class="row">
<article>
<h2>Article Section</h2>
<p>An article typically contains standalone content like a blog post or a news article.</p>
<figure>
<img src="https://www.tutorialkart.com/img/hummingbird.png" alt="Sample Image">
<figcaption>Figure: A humming bird</figcaption>
</figure>
</article>
<aside>
<h2>Related Links</h2>
<ul>
<li><a href="https://www.tutorialkart.com/html/">HTML Tutorials</a></li>
<li><a href="https://www.tutorialkart.com/css/">CSS Tutorials</a></li>
<li><a href="https://www.tutorialkart.com/javascript/">JavaScript Basics</a></li>
</ul>
</aside>
</div>
</main>
<footer>
<p>© 2024 HTML5 Demo Page. All rights reserved.</p>
</footer>
</body>
</html>
OUTPUT
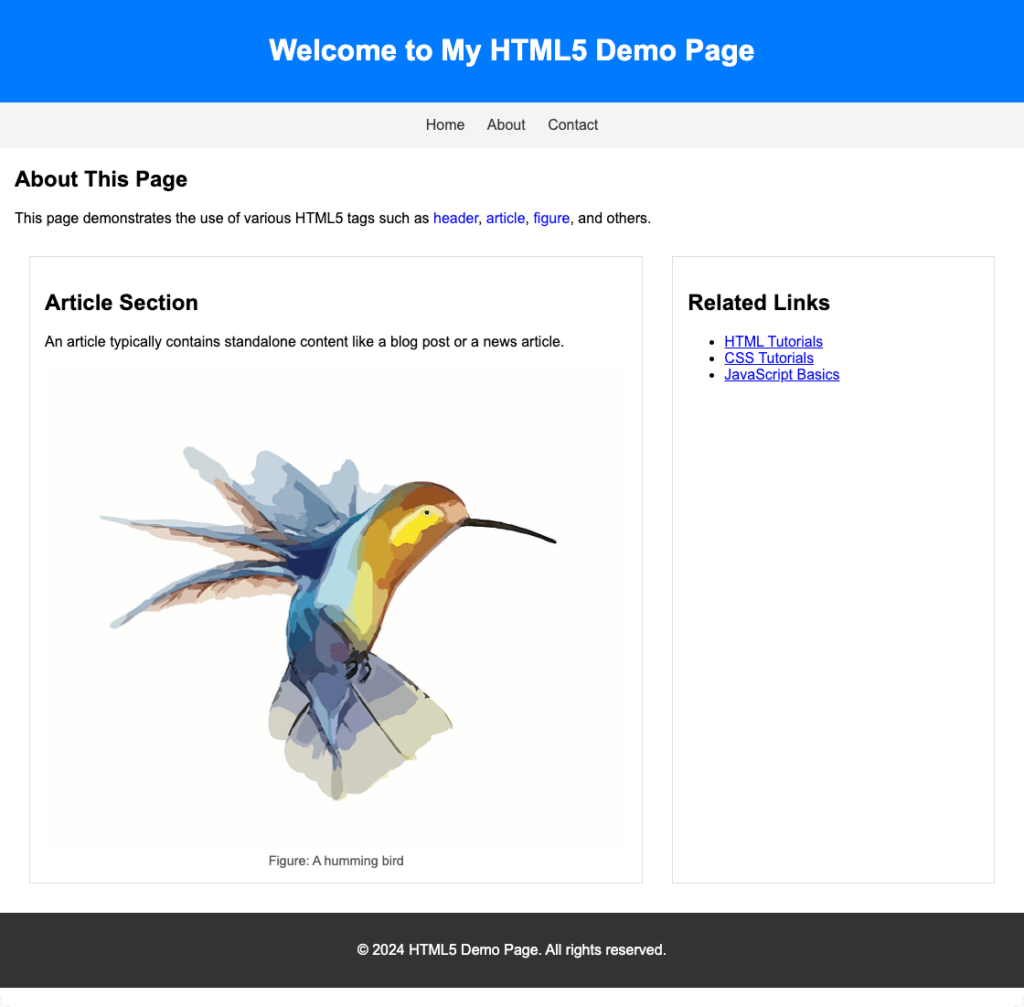
Explanation of Tags Used:
- <header>: Contains the page’s title or introductory content.
- <nav>: Navigation links to different parts of the site.
- <main>: The main content area.
- <section>: Groups related content in a logical block.
- <article>: Represents independent content, such as a blog post or article.
- <aside>: Contains supplementary content, like related links or ads.
- <figure>: Used to display images with optional captions.
- <figcaption>: Provides captions for the figure.
- <footer>: Displays footer information like copyright or links.
- <div>: Generic container used to group elements for styling or layout.
- <span>: Inline container used to apply styles or classes to text.
b. Write an HTML program to embed audio and video into an HTML web page.
PROCEDURE STEPS:
Step 1: Open the Visual Studio Code and create a HTML file named index.html file. We will embed a sample MP3 audio, and a YouTube Video into the HTML file.
Step 2: Locate the HTML file in File Explorer.
Step 3: Open the HTML in a Web Browser.
SOURCE CODE
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Embed Audio and Video</title>
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
line-height: 1.6;
}
h1, h2 {
color: #333;
}
.container {
max-width: 800px;
margin: 0 auto;
text-align: center;
}
.video-container {
margin: 20px 0;
}
.audio-container {
margin: 20px 0;
}
</style>
</head>
<body>
<div class="container">
<h1>Embed Audio and Video in HTML</h1>
<!-- Embedding Audio -->
<div class="audio-container">
<h2>Audio Example</h2>
<p>Listen to this sample audio:</p>
<audio controls>
<source src="https://www.tutorialkart.com/wp-content/uploads/2024/12/sample-audio.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
</div>
<!-- Embedding YouTube Video -->
<div class="video-container">
<h2>YouTube Video Example</h2>
<p>Watch this embedded YouTube video:</p>
<iframe width="560" height="315" src="https://www.youtube.com/embed/WegPzeDArUc?si=1cexGsjUdYEhwyFA" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture; web-share" referrerpolicy="strict-origin-when-cross-origin" allowfullscreen></iframe>
</div>
</div>
</body>
</html>
OUTPUT
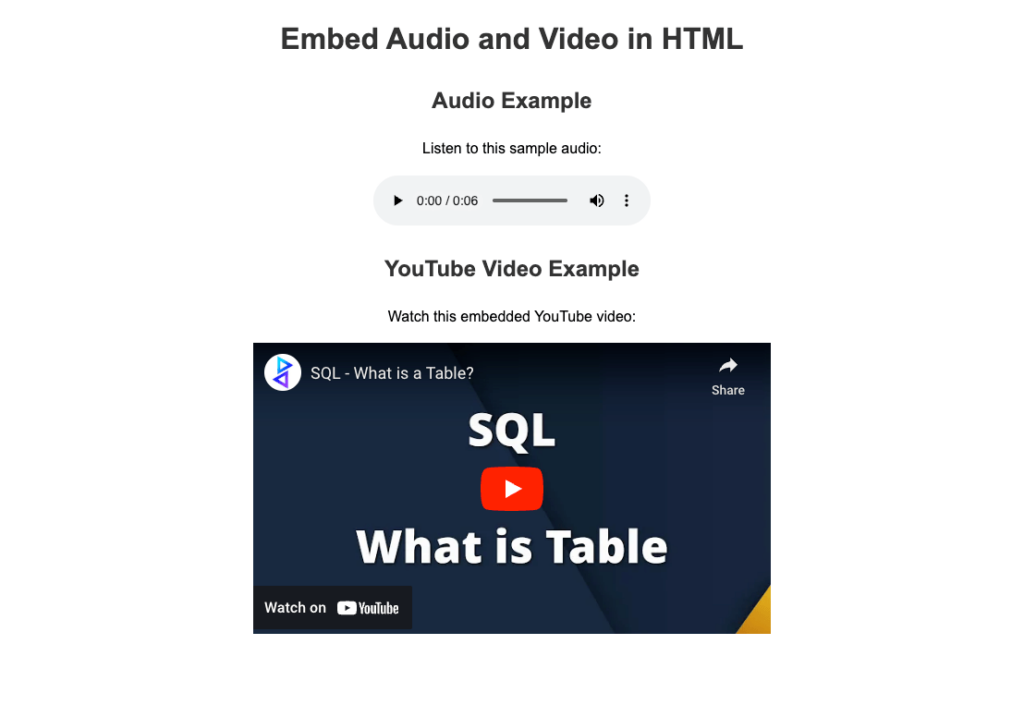
Explanation:
- Audio Embedding:
- The <audio> element is used to embed an audio file.
- The
controls
attribute adds play, pause, and volume controls. <source>
specifies the audio file (sample-audio.mp3
). Replace it with the actual path to your audio file.
- YouTube Video Embedding:
- The <iframe> tag is used to embed the YouTube video.
- Replace the
src
attribute value (https://www.youtube.com/embed/dQw4w9WgXcQ
) with the URL of the YouTube video you want to embed.
Key Points:
- Ensure the audio file (
sample-audio.mp3
) is in the same folder as your HTML file, or use the correct path. - The YouTube video can be replaced with any other video by updating the
src
attribute with the appropriate link. - This program is fully functional in any modern browser.
c. Write a program to apply different types of CSS — inline, internal, external styles to HTML elements.
PROCEDURE STEPS:
Step 1: Open the Visual Studio Code and create a HTML file named index.html file. Create the external CSS file as styles.css in the same location as that of index.html. Ensure both files are in the same directory.
Step 2: Locate the HTML file in File Explorer.
Step 3: Open the HTML in a Web Browser.
SOURCE CODE
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Styling Demonstration</title>
<!-- Linking External CSS -->
<link rel="stylesheet" href="styles.css">
<!-- Internal CSS -->
<style>
/* Internal CSS */
header {
background-color: #333;
color: white;
text-align: center;
padding: 10px;
}
footer {
background-color: #007BFF;
color: white;
text-align: center;
padding: 10px;
margin-top: 20px;
}
p.note {
color: #008800;
}
</style>
</head>
<body>
<!-- Inline CSS -->
<header>
<h1 style="font-size: 2.5rem; font-weight: bold;">CSS Styling Demo</h1>
</header>
<div>
<h2>Inline CSS Example</h2>
<p style="color: red; font-weight: bold;">
This paragraph is styled using inline CSS. Inline styles are defined directly in the HTML tag.
</p>
</div>
<div>
<h2>Internal CSS Example</h2>
<p class="note">
This paragraph is styled using internal CSS. Internal styles are defined in the <style> block within the <head> section.
</p>
</div>
<div>
<h2>External CSS Example</h2>
<p class="abcd">
This paragraph is styled using external CSS. External styles are defined in a separate CSS file and linked using the <link> tag.
</p>
</div>
<footer>
<p>© 2024 CSS Styling Demo</p>
</footer>
</body>
</html>
styles.css
/* External CSS */
body {
font-family: Arial, sans-serif;
margin: 20px;
line-height: 1.6;
background-color: #f9f9f9;
}
h1 {
color: #007BFF;
text-align: center;
}
p {
color: #333;
font-size: 1rem;
}
p.abcd {
color: blue;
}
div {
border: 1px solid #ddd;
padding: 10px;
background-color: #fff;
margin-bottom: 10px;
}
OUTPUT
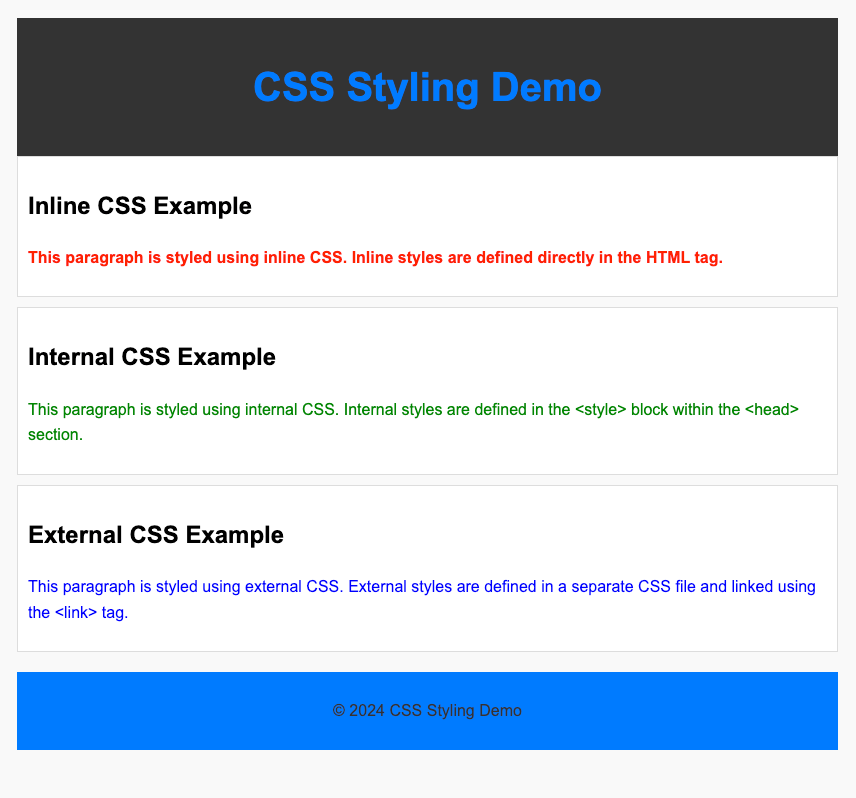
Explanation:
- Inline CSS:
- Directly added to an element using the
style
attribute. - Example:
<h1 style="font-size: 2.5rem; font-weight: bold;">
.
- Directly added to an element using the
- Internal CSS:
- Added within a
<style>
tag in the<head>
section of the HTML document. - Example:
header
andfooter
styles are defined internally.
- Added within a
- External CSS:
- Written in a separate file (e.g.,
styles.css
) and linked using<link>
in the<head>
section. - Example: The
body
,h1
,p
, anddiv
styles are applied fromstyles.css
.
- Written in a separate file (e.g.,