EXPERIMENT 4: Selector Forms
a. Write a program to apply different types of selector forms
- Simple selector (element, id, class, group, universal)
- Combinator selector (descendant, child, adjacent sibling, general sibling)
- Pseudo-class selector
- Pseudo-element selector
- Attribute selector
PROCEDURE STEPS:
Step 1: Open the Visual Studio Code and create a HTML file named index.html file. Write HTML content with <h1>, <div>, <p>, <form>, <input>, <ul>, <li>, and <a> tags. Use class and id attributes for these elements.
Step 2: Locate the HTML file in File Explorer.
Step 3: Open the HTML in a Web Browser.
SOURCE CODE
index.html
</>
Copy
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CSS Selectors Experiment</title>
<style>
/* Simple Selectors */
/* Selects all <p> elements and sets their text color to blue */
p {
color: blue;
padding: 10px;
}
/* Selects the element with ID 'unique' and makes its font bold */
#unique {
font-weight: bold;
}
/* Selects all elements with the class 'highlight' and gives them a yellow background */
.highlight {
background-color: yellow;
border-radius: 10px;
}
/* Selects all <div> and <span> elements and adds a 5px margin */
div, span {
margin: 5px;
}
/* Applies Arial font-family to all elements */
* {
font-family: Arial, sans-serif;
}
/* Combinator Selectors */
/* Selects all <p> elements inside a <div>, regardless of nesting level, and sets their color to green */
div p {
color: green;
}
/* Selects all <p> elements that are direct children of a <div> and adds a black border */
div > p {
border: 1px solid black;
}
/* Selects the first <p> element immediately following an <h1> and increases its font size */
h1 + p {
font-size: 20px;
}
/* Selects all <p> elements that are siblings of an <h1>, appearing anywhere after it, and changes their color to red */
h1 ~ p {
color: red;
}
/* Pseudo-class Selectors */
/* Changes the color of a link when the user hovers over it */
a:hover {
color: orange;
}
/* Highlights input fields with a blue outline when they are focused */
input:focus {
outline: 2px solid blue;
}
/* Alternates background colors for odd list items */
li:nth-child(odd) {
background-color: #f0f0f0;
}
/* Alternates background colors for even list items */
li:nth-child(even) {
background-color: #d0f0d0;
}
/* Pseudo-element Selectors */
/* Styles the first line of every <p> element to be bold */
p::first-line {
font-weight: bold;
}
/* Adds a bullet point before every <p> element's content inside a div */
div p::before {
content: "\2022 ";
color: gray;
}
/* Styles All Forms */
form {
padding: 1em;
border: 1px solid #eee;
width: fit-content;
}
/* Attribute Selectors */
/* Selects input elements of type 'text' and adds a gray border */
[type="text"] {
border: 2px solid gray;
padding: 5px;
border-radius: 10px;
}
/* Selects input elements of type 'button' and styles them with a light blue background and padding */
[type="button"] {
background-color: lightblue;
padding: 5px;
}
</style>
</head>
<body>
<h1>CSS Selector Demonstration</h1>
<div id="unique">
<p>This paragraph is inside a div and styled using a descendant selector.</p>
<p class="highlight">This paragraph is styled with a class and a child selector.</p>
</div>
<h1>Another Heading</h1>
<p>This paragraph comes right after an h1 and is styled with an adjacent sibling selector.</p>
<p>This is another paragraph styled with a general sibling selector.</p>
<ul>
<li>List Item 1</li>
<li>List Item 2</li>
<li>List Item 3</li>
<li>List Item 4</li>
</ul>
<hr>
<h3>My Form</h3>
<form>
<p>Sample Form</p>
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<br><br>
<input type="button" id="submit" value="Submit">
</form>
<br>
<a href="#">Hover over this link</a>
<script>
// JavaScript to show hover effects dynamically
document.getElementById('submit').addEventListener('click', function () {
alert('Button clicked!');
});
</script>
</body>
</html>
OUTPUT
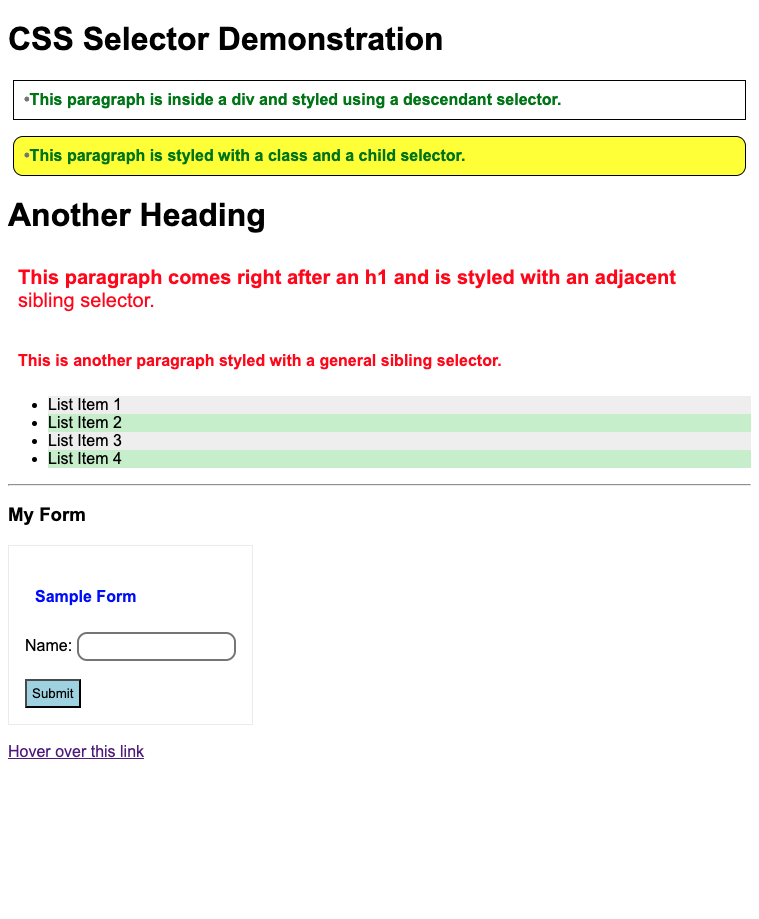