- Applying JavaScript – internal and external, I/O, Type Conversion
a. Write a program to embed internal and external JavaScript in
a web page.
b. Write a program to explain the different ways for displaying output.
c. Write a program to explain the different ways for taking input.
d. Create a webpage which uses prompt dialogue box to ask a voter for his name and age.
Display the information in table format along with either the voter can vote or not
a. Write a program to embed internal and external JavaScript in
a web page.
Steps to Implement
- Create the HTML file (
index.html
). - Write Internal JavaScript inside
<script>
tags. - Create an External JavaScript file (
script.js
) and link it.
index.html
</>
Copy
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Embed Internal and External JavaScript</title>
<script>
// Internal JavaScript
function internalMessage() {
alert("Hello from Internal JavaScript!");
}
</script>
</head>
<body>
<h1>JavaScript Embedding Example</h1>
<button onclick="internalMessage()">Click for Internal JS</button>
<button onclick="externalMessage()">Click for External JS</button>
<!-- Linking External JavaScript -->
<script src="script.js"></script>
</body>
</html>
script.js
</>
Copy
// External JavaScript
function externalMessage() {
alert("Hello from External JavaScript!");
}
Output
- Clicking the first button shows an alert:
"Hello from Internal JavaScript!"
- Clicking the second button shows an alert:
"Hello from External JavaScript!"
Click on the button ‘Click for Internal JS’
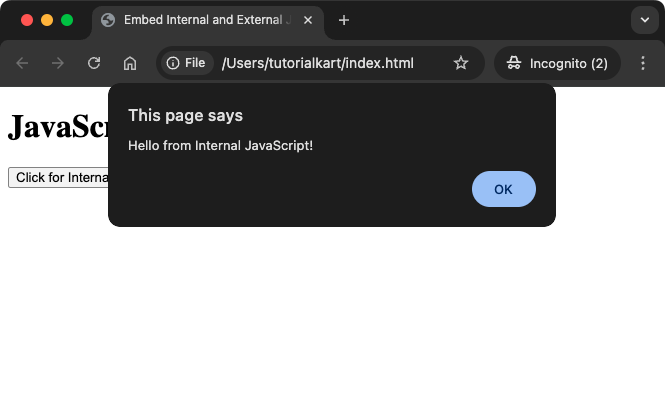
Click on the button ‘Click for External JS’
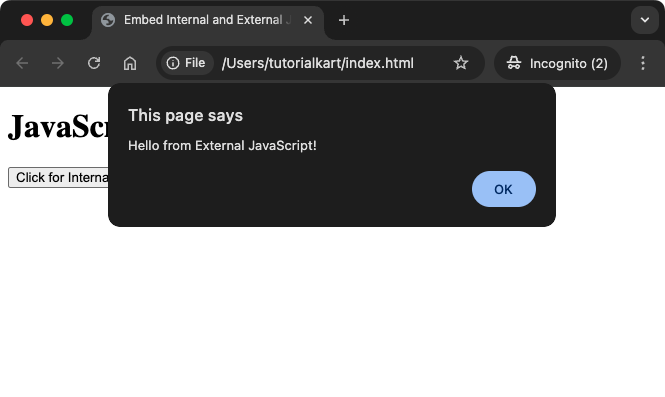
b. Write a program to explain the different ways for displaying output.
Steps to Implement
- Create the HTML file (
index.html
). - Write Internal JavaScript inside
<script>
tags.
index.html
</>
Copy
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Output Methods</title>
</head>
<body>
<h1>JavaScript Output Methods</h1>
<button onclick="showAlert()">Show Alert</button>
<button onclick="writeToDocument()">Write to Document</button>
<button onclick="updateInnerHTML()">Update HTML</button>
<button onclick="logToConsole()">Log to Console</button>
<p id="output">This text will be updated.</p>
<script>
// 1. Display output using alert()
function showAlert() {
alert("This is an alert box!");
}
// 2. Display output using document.write()
function writeToDocument() {
document.write("This text is written using document.write()");
}
// 3. Display output using innerHTML
function updateInnerHTML() {
document.getElementById("output").innerHTML = "Updated using innerHTML!";
}
// 4. Display output using console.log()
function logToConsole() {
console.log("This is a console message.");
}
</script>
</body>
</html>
Output
- Clicking “Show Alert” → Displays an alert box.
- Clicking “Write to Document” → Overwrites the entire page with a message.
- Clicking “Update HTML” → Updates the
<p>
element text. - Clicking “Log to Console” → Prints a message in the browser console.
When you click on Show Alert button.

When you click on Write to Document button.

When you click on Update HTML button.

When you click on Log to Console button.

c. Write a program to explain the different ways for taking input.
Steps to Implement
- Create the HTML file (
index.html
). - Write Internal JavaScript inside
<script>
tags.
index.html
</>
Copy
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Input Methods</title>
</head>
<body>
<h1>JavaScript Input Methods</h1>
<button onclick="usePrompt()">Take Input using Prompt</button>
<button onclick="useConfirm()">Take Input using Confirm</button>
<br><br>
<!-- Input field -->
<label for="userInput">Enter your name: </label>
<input type="text" id="userInput" placeholder="Type something">
<button onclick="getInputValue()">Submit</button>
<p id="output"></p>
<script>
// 1. Taking input using prompt()
function usePrompt() {
let name = prompt("Enter your name:");
if (name) {
document.getElementById("output").innerHTML = "You entered: " + name;
} else {
document.getElementById("output").innerHTML = "You did not enter anything.";
}
}
// 2. Taking input using an HTML input field
function getInputValue() {
let inputValue = document.getElementById("userInput").value;
document.getElementById("output").innerHTML = "Input value: " + inputValue;
}
// 3. Taking input using confirm()
function useConfirm() {
let response = confirm("Do you like JavaScript?");
if (response) {
document.getElementById("output").innerHTML = "You clicked OK! 😊";
} else {
document.getElementById("output").innerHTML = "You clicked Cancel. 😢";
}
}
</script>
</body>
</html>
Output
Clicking “Take Input using Prompt” → Shows a popup input box.

Clicking “Submit” after entering text in the input field → Displays the value on the page.

Clicking “Take Input using Confirm” → Displays "OK"
or "Cancel"
response.

d. Create a webpage which uses prompt dialogue box to ask a voter for his name and age.
Display the information in table format along with either the voter can vote or not
Steps to Implement
- Create the HTML file (
index.html
). - Write Internal JavaScript inside
<script>
tags.
index.html
</>
Copy
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Voter Eligibility Check</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
margin: 50px;
}
table {
margin: 20px auto;
border-collapse: collapse;
width: 50%;
}
th, td {
border: 1px solid black;
padding: 10px;
text-align: center;
}
th {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<h1>Voter Eligibility Checker</h1>
<script>
// Ask user for Name and Age
let name = prompt("Enter your name:");
let age = prompt("Enter your age:");
// Convert age to number
age = Number(age);
// Determine voter eligibility
let eligibility = (age >= 18) ? "Eligible to Vote ✅" : "Not Eligible to Vote ❌";
// Display the result in a table format
if (name && !isNaN(age)) {
document.write(`
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>Voting Eligibility</th>
</tr>
<tr>
<td>${name}</td>
<td>${age}</td>
<td>${eligibility}</td>
</tr>
</table>
`);
} else {
document.write("<p style='color:red;'>Invalid input. Please refresh and enter valid details.</p>");
}
</script>
</body>
</html>
Output

Enter your name and click OK.


Enter your age, and click OK.

