Changing Background Color of a Link on Hover Using CSS
Changing the background color of a link on hover enhances user experience by providing visual feedback when users interact with links. This technique is widely used in navigation menus, buttons, and interactive elements to make them more noticeable.
The CSS :hover
pseudo-class allows you to change the background color of a link (<a>
tag) when the user hovers over it. Below is a basic example of how to achieve this effect:
/* Change background color on hover */
a:hover {
background-color: yellow;
}
Examples
Example 1. Changing Background Color of a Link on Hover
In this example, we change the background color of the link to yellow when the user hovers over it.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
a {
text-decoration: none;
color: blue;
padding: 5px;
}
a:hover {
background-color: yellow;
}
</style>
</head>
<body>
<p><a href="https://www.example.com">Hover Over Me</a></p>
</body>
</html>
Output:
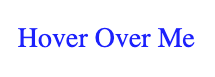
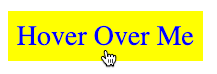
Example 2. Changing Background and Font Color on Hover
In this example, we change both the background color and the font color when the user hovers over the link.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
a {
text-decoration: none;
color: white;
background-color: blue;
padding: 5px;
border-radius: 3px;
}
a:hover {
background-color: red;
color: yellow;
}
</style>
</head>
<body>
<p><a href="https://www.example.com">Hover Over Me</a></p>
</body>
</html>
Output:
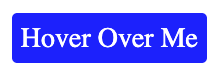
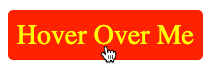
Example 3. Changing Background Color for a Specific Link
Sometimes, you may want to change the hover background color only for a specific link. You can achieve this by targeting links inside a specific class.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
a {
text-decoration: none;
color: black;
padding: 5px;
}
.custom-link:hover {
background-color: green;
color: white;
}
</style>
</head>
<body>
<p><a href="https://www.example.com">Regular Link</a></p>
<p><a class="custom-link" href="https://www.example.com">Hover Over Me (Custom Link)</a></p>
</body>
</html>
Output:
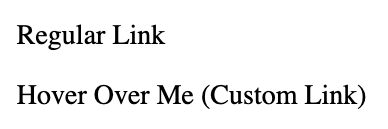
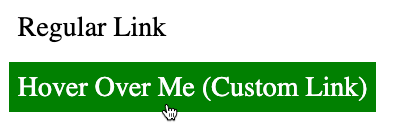
Conclusion
Using the :hover
pseudo-class to change the background color of links is a great way to improve user interaction.