Setting Background Color of a Link Using CSS
Changing the background color of a link using CSS enhances its visibility and improves user interaction. This technique is useful for navigation menus, call-to-action buttons, and highlighted links.
The CSS background-color
property allows you to set a custom background for a link (<a>
tag). Below is a basic example:
/* Set background color for all links */
a {
background-color: yellow;
padding: 5px;
text-decoration: none;
color: black;
}
Examples
Example 1. Setting a Background Color for a Link
In this example, we set a yellow background for links while removing the underline.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
a {
background-color: yellow;
padding: 5px;
text-decoration: none;
color: black;
}
</style>
</head>
<body>
<p><a href="https://www.example.com">Visit Example</a></p>
</body>
</html>
Output:
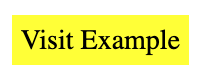
Example 2. Changing Background Color on Hover
In this example, we change the background color when the user hovers over the link.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
a {
background-color: lightblue;
padding: 5px;
text-decoration: none;
color: black;
transition: background-color 0.3s ease;
}
a:hover {
background-color: blue;
color: white;
}
</style>
</head>
<body>
<p><a href="https://www.example.com">Hover Over Me</a></p>
</body>
</html>
Output:
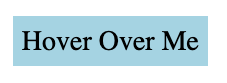
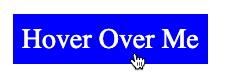
Example 3. Styling a Link to Look Like a Button
In this example, we create a button-like link with a background color, padding, and rounded corners.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<style>
a.button {
display: inline-block;
background-color: green;
color: white;
padding: 10px 20px;
text-decoration: none;
border-radius: 5px;
font-size: 16px;
font-weight: bold;
text-align: center;
transition: background-color 0.3s ease;
}
a.button:hover {
background-color: darkgreen;
}
</style>
</head>
<body>
<p><a href="https://www.example.com" class="button">Click Me</a></p>
</body>
</html>
Output:
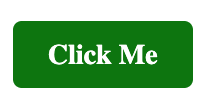
Conclusion
Using the background-color
property, you can easily customize links to improve visibility and enhance user interaction. You can also apply hover effects and style links as buttons to create a better user experience.