What is a Binary Tree?
A binary tree is a hierarchical data structure in which each node has at most two children, commonly referred to as the left child and the right child.
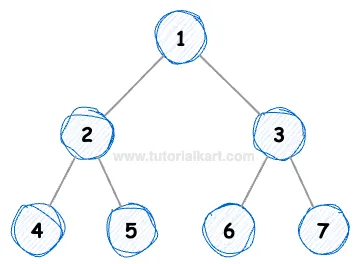
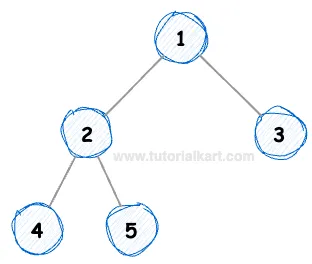
It is used to represent data in a structured and easily accessible way, making it fundamental for many algorithms in computer science such as searching, sorting, and expression parsing.
Properties of Binary Trees
Binary trees have several important properties that are essential to understanding how they work:
- Node Structure: Each node contains data and pointers (or references) to its left and right children.
- Root Node: The topmost node of the tree, which acts as the entry point to the tree.
- Leaf Nodes: Nodes that do not have any children. These represent the end points of the tree.
- Subtrees: Every node in a binary tree can serve as the root of its own subtree, which is itself a binary tree.
- Height (or Depth): The height of a binary tree is the length of the longest path from the root to a leaf node.
- Balance: A balanced binary tree is one where the left and right subtrees of every node differ in height by no more than one, which helps in maintaining efficient operations.
Types of Binary Trees
There are several types of binary trees, each with distinct characteristics and use cases:
- Full Binary Tree: Every node, except for the leaf nodes, has exactly two children. This structure is ideal when a uniform tree shape is needed.
- Complete Binary Tree: All levels are fully filled except possibly the last, which is filled from left to right. This type of tree is often used in heap data structures.
- Skewed Binary Tree: A tree where every node has only one child, either left or right. This results in a structure similar to a linked list and can be classified as left-skewed or right-skewed.
- Balanced Binary Tree: A tree that maintains its height by ensuring the difference in height between the left and right subtrees of any node is minimal. Examples include AVL trees and Red-Black trees, which help maintain efficient search, insertion, and deletion operations.
Understanding these types of binary trees will help you choose the most appropriate structure based on your specific application requirements, whether for simple data storage or more complex operations like balancing and efficient searching.