Complete Binary Tree
A complete binary tree is a binary tree in which every level, except possibly the last, is completely filled, and all nodes are as far left as possible.
This tutorial directly addresses the concept of a complete binary tree, provides detailed examples with array notations and graphical diagrams, and explains why specific trees meet or do not meet the complete binary tree criteria. We will also discuss some applications of complete binary trees.
Definition of Complete Binary Tree
A complete binary tree is defined as a binary tree in which all levels are completely filled except possibly the last level, which is filled from left to right without any gaps. In an array representation, there should be no empty spots between the elements up to the last element.
Example 1: Complete Binary Tree (Fully Filled)
In this example, we represent a complete binary tree using an array notation where every level is completely filled. The array is: [1, 2, 3, 4, 5, 6, 7].
Graphical Diagram Representation:
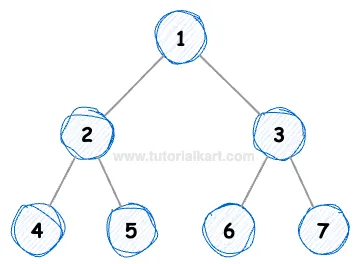
Explanation:
- The root node is
1
at index 0. - The children of
1
are2
(index 1) and3
(index 2). - The children of
2
are4
(index 3) and5
(index 4). - The children of
3
are6
(index 5) and7
(index 6). - All levels are completely filled, which satisfies the complete binary tree criteria.
Example 2: Incomplete Binary Tree (Not Complete)
In this example, we examine an array representation that does not meet the complete binary tree criteria. Consider the array: [1, 2, 3, 4, -, 6, 7] where -
indicates a missing node.
Graphical Diagram Representation:
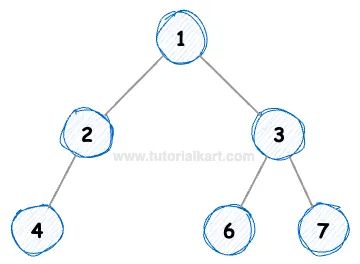
Explanation:
- The root node is
1
at index 0 with children2
(index 1) and3
(index 2). - For node
2
at index 1, the left child is4
(index 3), but the right child is missing (indicated by-
at index 4). - Even though node
3
at index 2 has valid children (6
and7
), the gap at node2
violates the left-to-right filling rule for the last level. - Thus, this tree is not complete because there is a missing node in the expected left-to-right order.
Example 3: Complete Binary Tree (Last Level Partially Filled)
In this example, we consider a tree that is complete even though the last level is not fully filled. The array is: [1, 2, 3, 4, 5].
Graphical Diagram Representation:
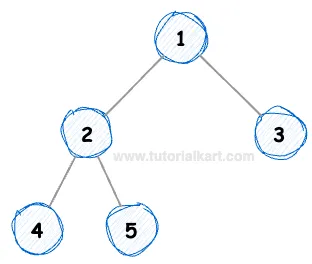
Explanation:
- The root node
1
is at index 0, with children2
(index 1) and3
(index 2). - For node
2
at index 1, the left child is4
(index 3) and the right child is5
(index 4). - Node
3
at index 2 does not have any children, which is acceptable because the last level may be partially filled, as long as nodes are filled from left to right. - This tree is complete because all levels except the last are fully filled and the last level has no gaps from the left.
Example 4: Complete Binary Tree with Minimal Last Level
In this final example, we represent a complete binary tree where the last level has only one node. The array representation is: [1, 2, 3, 4].
Graphical Diagram Representation:
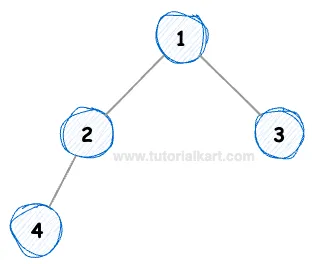
Explanation:
- The root node is
1
at index 0, with children2
(index 1) and3
(index 2). - For node
2
at index 1, the left child is4
at index 3, and no right child exists. - This arrangement is acceptable because in a complete binary tree, the last level does not need to be full as long as nodes are filled from left to right.
- The tree satisfies the complete binary tree properties.
Applications of Complete Binary Trees
Complete binary trees are particularly useful in implementing binary heaps, which are commonly used in priority queues and heap sort algorithms. Their predictable and balanced structure allows for efficient insertion, deletion, and access operations, making them ideal for performance-critical applications such as task scheduling and memory management.
Conclusion
In this tutorial, we defined a complete binary tree and explored various examples using array notations and graphical diagrams. We demonstrated what makes a binary tree complete and identified scenarios where a tree is not complete. Finally, we discussed the practical applications of complete binary trees in computer science. Understanding these concepts is fundamental for working with data structures like heaps and for optimizing algorithm performance.