Representation of Binary Trees
Representation of binary trees refers to the way in which the structure of a binary tree is stored in memory. There are two common representations: using pointers (linked representation) and using arrays (sequential representation). Each method has its own advantages and disadvantages, and the choice depends on the specific requirements of the application.
Binary Trees using Linked Representation
This method involves creating a node structure where each node contains data along with pointers to its left and right children.
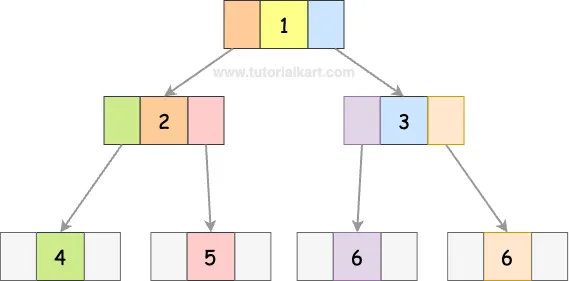
This linked representation is dynamic, allowing the tree to grow and shrink as needed.
Advantages:
- Dynamic memory allocation – nodes are created as needed.
- Efficient use of memory for sparse trees.
- Easy insertion and deletion of nodes.
Disadvantages:
- Requires extra memory for storing pointers.
- Potential overhead due to dynamic memory management.
- Cache performance can be less optimal compared to array representation.
Using Arrays (Sequential Representation)
In the array representation, a binary tree is stored in a contiguous block of memory, typically an array.
[1, 2, 3, 4, 5, 6, 7]
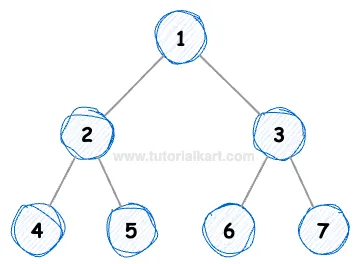
For a complete binary tree, the root is stored at index 0 (or 1, depending on the convention), the left child of the node at index i
is at 2*i + 1
and the right child is at 2*i + 2
(for 0-indexed arrays).
Advantages:
- Simpler implementation when the tree is complete or nearly complete.
- Efficient random access due to contiguous memory allocation.
- No overhead of pointer storage.
Disadvantages:
- Wastes memory if the tree is sparse.
- Fixed size – difficult to expand dynamically.
- Insertion and deletion operations can be inefficient.
Which Representation is Used When?
The choice of representation depends on the characteristics of the binary tree and the operations required:
- Linked Representation (Pointers): Ideal for trees that are sparse or require frequent insertions and deletions. It offers flexibility in memory usage and dynamic structure modifications.
- Sequential Representation (Arrays): Best suited for complete or nearly complete binary trees where efficient random access is necessary, and memory overhead is a concern.
Which is the Most Common Representation?
For most applications involving binary trees, the linked representation using pointers is the most common. This is due to its dynamic nature and flexibility, which make it well-suited for a wide variety of tree operations. However, when working with complete binary trees such as heaps, the array representation is often preferred due to its efficient indexing and memory utilization.