Go – Array Functions
In Go, arrays are fixed-size collections of elements of the same type. You can use functions to perform operations on arrays, such as iterating, updating, or performing calculations. Functions can accept arrays as arguments, return arrays, or modify array elements.
In this tutorial, we will explore how to create and use functions for working with arrays in Go, with practical examples and detailed explanations.
Syntax for Array Functions
The syntax to define a function that works with arrays is:
</>
Copy
func functionName(array [length]elementType) returnType {
// Function body
}
Here:
functionName
: The name of the function.array
: The parameter representing the array passed to the function.length
: The fixed size of the array.elementType
: The type of elements in the array (e.g.,int
,float64
, etc.).returnType
: The type of the value returned by the function.
Examples of Array Functions
1 Function to Print Array Elements
This function takes an array as input and prints its elements:
</>
Copy
package main
import "fmt"
// Function to print array elements
func printArray(arr [5]int) {
for i, v := range arr {
fmt.Printf("Element at index %d: %d\n", i, v)
}
}
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// Call the function
printArray(numbers)
}
Explanation
- Define Function: The function
printArray
accepts an array of 5 integers as input. - Iterate and Print: A
for
loop withrange
is used to iterate through the array and print each element. - Call Function: The array
numbers
is passed to the function for printing.
Output
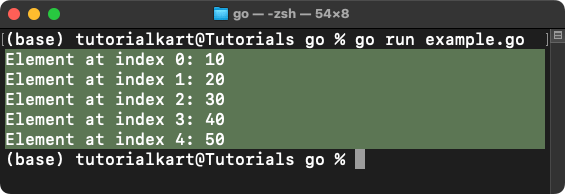
2 Function to Find the Sum of Array Elements
This function calculates the sum of all elements in an array:
</>
Copy
package main
import "fmt"
// Function to calculate the sum of array elements
func sumArray(arr [5]int) int {
sum := 0
for _, v := range arr {
sum += v
}
return sum
}
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// Call the function and print the result
fmt.Println("Sum of array elements:", sumArray(numbers))
}
Explanation
- Define Function: The function
sumArray
accepts an array of 5 integers and returns their sum. - Iterate and Sum: A
for
loop withrange
iterates through the array, adding each element tosum
. - Return Result: The sum is returned and printed in the
main
function.
Output
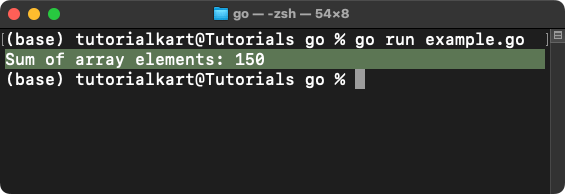
3 Function to Reverse an Array
This function reverses the order of elements in an array:
</>
Copy
package main
import "fmt"
// Function to reverse an array
func reverseArray(arr [5]int) [5]int {
var reversed [5]int
for i, v := range arr {
reversed[len(arr)-1-i] = v
}
return reversed
}
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// Call the function and print the result
fmt.Println("Reversed Array:", reverseArray(numbers))
}
Explanation
- Define Function: The function
reverseArray
accepts an array of 5 integers and returns a reversed array. - Reverse Logic: A
for
loop assigns elements from the input array to the reversed array in reverse order. - Return Result: The reversed array is returned and printed in the
main
function.
Output
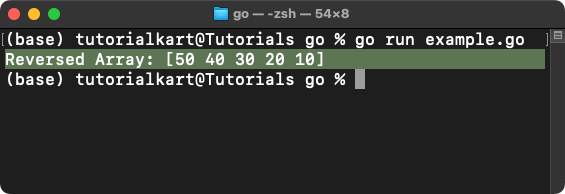
Points to Remember
- Fixed Size: Arrays passed to functions must have a fixed size matching the parameter definition.
- Pass by Value: Arrays in Go are passed by value, meaning the original array is not modified unless a pointer is used.
- Return Types: Functions can return arrays, which can be assigned to variables or directly used.
- Utility: Array functions are useful for modularizing repetitive tasks, such as calculations and transformations.