Go – Array of Floats
Arrays in Go are fixed-size collections of elements of the same type. An array of floats is a collection where each element is of the type float64
.
In this tutorial, we will cover how to create and perform basic CRUD (Create, Read, Update, Delete) operations on arrays of floats with practical examples and detailed explanations.
Syntax for Array of Floats
The syntax to declare an array of floats is as follows:
</>
Copy
var arrayName [length]float64
Here:
arrayName
: The name of the array.length
: The fixed size of the array (number of elements).float64
: The type of elements in the array.
Basic CRUD Operations on Float Arrays
1. Create an Array of Floats
Here’s how to create and initialize an array of floats:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
var numbers [4]float64
numbers[0] = 1.1
numbers[1] = 2.2
numbers[2] = 3.3
numbers[3] = 4.4
// Print the array
fmt.Println("Array of Floats:", numbers)
}
Explanation
- Declare Array: The array
numbers
is declared with a fixed size of 4 and element typefloat64
. - Assign Values: Elements are assigned float values using their indices.
- Print Array: The entire array is printed using
fmt.Println
.
Output
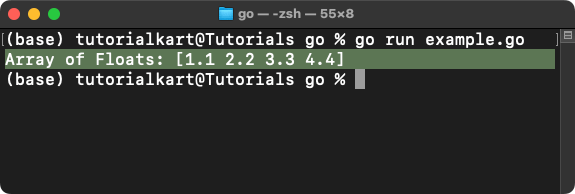
2. Read Elements from an Array
Access array elements using their indices:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [4]float64{1.1, 2.2, 3.3, 4.4}
// Access and print individual elements
fmt.Println("First Element:", numbers[0])
fmt.Println("Third Element:", numbers[2])
}
Explanation
- Access Elements: Array elements are accessed using zero-based indexing.
- Print Elements: Specific elements are printed using their indices.
Output
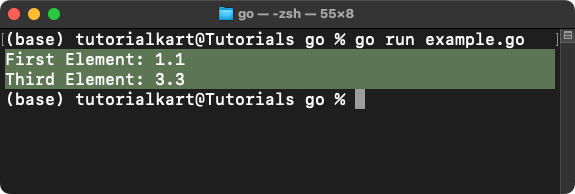
3. Update Elements in an Array
Update an element in an array by assigning a new value to its index:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [4]float64{1.1, 2.2, 3.3, 4.4}
// Update an element
numbers[2] = 9.9
// Print updated array
fmt.Println("Updated Array:", numbers)
}
Explanation
- Declare and Initialize: The array
numbers
is initialized with float values. - Update Element: The value at index
2
is updated to9.9
. - Print Updated Array: The modified array is printed using
fmt.Println
.
Output
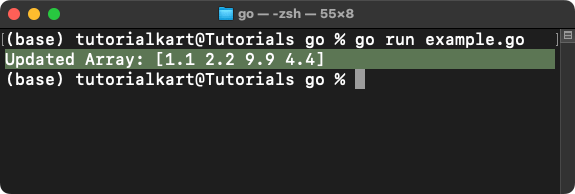
4. Delete Elements from an Array
To “delete” an element, set its value to the zero value for floats (0.0
):
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [4]float64{1.1, 2.2, 3.3, 4.4}
// "Delete" an element by setting it to zero
numbers[1] = 0.0
// Print updated array
fmt.Println("Array after deletion:", numbers)
}
Explanation
- Set Zero Value: The value at index
1
is set to0.0
, simulating deletion. - Print Array: The array with the updated value is printed using
fmt.Println
.
Output
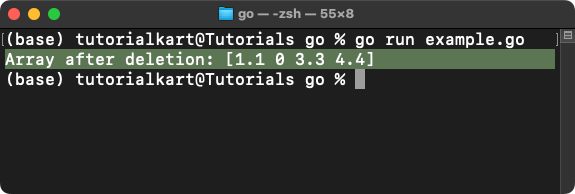
Points to Remember
- Fixed Size: Arrays in Go have a fixed size defined during declaration.
- Zero-Based Indexing: Array elements are accessed using indices starting from
0
. - Zero Value: Uninitialized elements in a float array default to
0.0
. - Static Collections: Arrays are ideal for scenarios where the size is known and constant.