Go – Array of Integers
Arrays in Go are fixed-size collections of elements of the same type. An array of integers is a collection where each element is of the type int
.
In this tutorial, we will cover how to create and perform basic CRUD (Create, Read, Update, Delete) operations on arrays of integers with practical examples and detailed explanations.
Syntax for Array of Integers
The syntax for declaring an array of integers is as follows:
</>
Copy
var arrayName [length]int
Here:
arrayName
: The name of the array.length
: The fixed size of the array (number of elements).int
: The type of elements in the array.
Basic CRUD Operations on Integer Arrays
1 Create an Array of Integers
Here’s how to create and initialize an array of integers:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
var numbers [5]int
numbers[0] = 10
numbers[1] = 20
numbers[2] = 30
numbers[3] = 40
numbers[4] = 50
// Print the array
fmt.Println("Array of Integers:", numbers)
}
Explanation
- Declare Array: The array
numbers
is declared with a fixed size of 5 and element typeint
. - Assign Values: Elements are assigned values using their indices.
- Print Array: The entire array is printed using
fmt.Println
.
Output
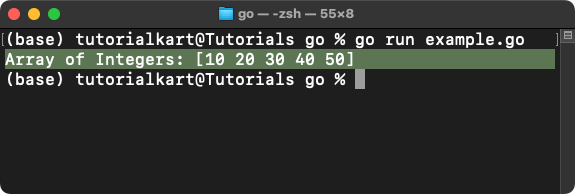
2 Read Elements from an Array
Access array elements using their indices:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// Access and print individual elements
fmt.Println("First Element:", numbers[0])
fmt.Println("Third Element:", numbers[2])
}
Explanation
- Access Elements: Array elements are accessed using zero-based indexing.
- Print Elements: Specific elements are printed using their indices.
Output
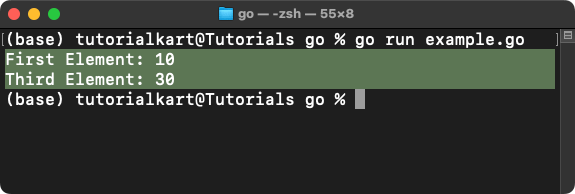
3 Update Elements in an Array
Update an element in an array by assigning a new value to its index:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// Update an element
numbers[2] = 99
// Print updated array
fmt.Println("Updated Array:", numbers)
}
Explanation
- Declare and Initialize: The array
numbers
is initialized with integer values. - Update Element: The value at index
2
is updated to99
. - Print Updated Array: The modified array is printed using
fmt.Println
.
Output
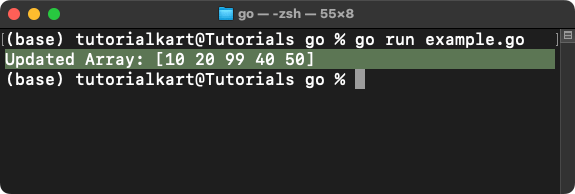
4 Delete Elements from an Array
To “delete” an element, set its value to the zero value for integers (0
):
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// "Delete" an element by setting it to zero
numbers[1] = 0
// Print updated array
fmt.Println("Array after deletion:", numbers)
}
Explanation
- Set Zero Value: The value at index
1
is set to0
, simulating deletion. - Print Array: The array with the updated value is printed using
fmt.Println
.
Output
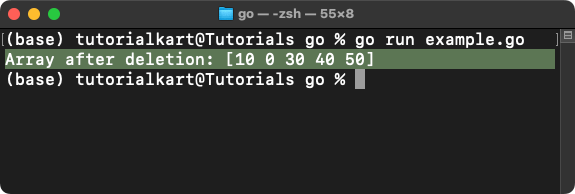
Points to Remember
- Fixed Size: Arrays in Go have a fixed size defined during declaration.
- Zero-Based Indexing: Array elements are accessed using indices starting from
0
. - Zero Value: Uninitialized elements in an integer array default to
0
. - Static Collections: Arrays are suitable for scenarios where the size is known and constant.