Go – Array of Maps
Arrays in Go are fixed-size collections of elements of the same type. An array of maps is a collection where each element is a map, allowing you to store key-value pairs in an organized structure.
In this tutorial, we will cover how to create and perform basic CRUD (Create, Read, Update, Delete) operations on arrays of maps with practical examples and detailed explanations.
Syntax for Array of Maps
The syntax to declare an array of maps involves using the map type as the element type:
</>
Copy
var arrayName [length]map[keyType]valueType
Here:
arrayName
: The name of the array.length
: The fixed size of the array.map[keyType]valueType
: The type of each map in the array, wherekeyType
is the type of the keys, andvalueType
is the type of the values.
Basic CRUD Operations on Array of Maps
1 Create an Array of Maps
Here’s how to create and initialize an array of maps:
</>
Copy
package main
import "fmt"
func main() {
// Declare an array of maps
var data [2]map[string]string
// Initialize each map
data[0] = map[string]string{"Name": "Alice", "Age": "25"}
data[1] = map[string]string{"Name": "Bob", "Age": "30"}
// Print the array
fmt.Println("Array of Maps:", data)
}
Explanation
- Declare Array: The array
data
is declared with a size of 2 and element typemap[string]string
. - Initialize Maps: Each map in the array is initialized with key-value pairs using the map literal syntax.
- Print Array: The entire array is printed using
fmt.Println
.
Output
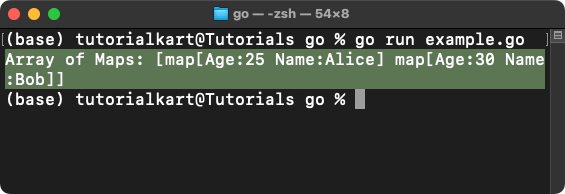
2 Read Elements from an Array
Access maps in the array using their indices and retrieve specific values:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array of maps
data := [2]map[string]string{
{"Name": "Alice", "Age": "25"},
{"Name": "Bob", "Age": "30"},
}
// Access and print specific values
fmt.Println("First Element:", data[0])
fmt.Println("Second Element's Name:", data[1]["Name"])
}
Explanation
- Access Elements: Array elements are accessed using indices, and map values are retrieved using their keys.
- Print Specific Fields: Specific fields from the maps are printed using their keys.
Output
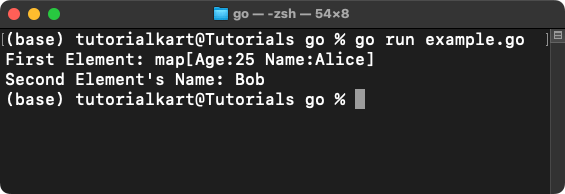
3 Update Elements in an Array
Update a value in one of the maps in the array:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array of maps
data := [2]map[string]string{
{"Name": "Alice", "Age": "25"},
{"Name": "Bob", "Age": "30"},
}
// Update a value in the second map
data[1]["Age"] = "31"
// Print updated array
fmt.Println("Updated Array:", data)
}
Explanation
- Update Value: The value associated with the key
"Age"
in the second map is updated to"31"
. - Print Updated Array: The modified array is printed using
fmt.Println
.
Output
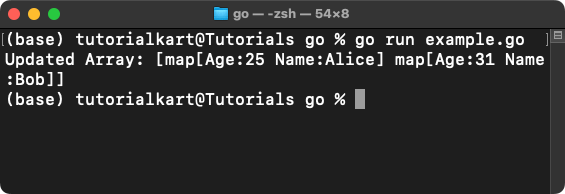
4 Delete Elements from an Array
To “delete” a map from the array, reset it to nil
or reinitialize it:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array of maps
data := [2]map[string]string{
{"Name": "Alice", "Age": "25"},
{"Name": "Bob", "Age": "30"},
}
// "Delete" the second map by resetting it to nil
data[1] = nil
// Print updated array
fmt.Println("Array after deletion:", data)
}
Explanation
- Reset Element: The second map in the array is reset to
nil
, effectively deleting it. - Print Updated Array: The updated array is printed using
fmt.Println
.
Output
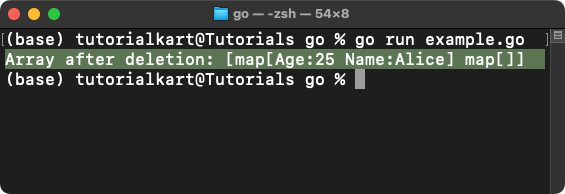
Points to Remember
- Fixed Size: Arrays in Go have a fixed size defined during declaration.
- Dynamic Maps: Maps within the array can grow or shrink dynamically.
- Nil Maps: Maps in the array need to be initialized before use; otherwise, they are
nil
. - Static Collections: Arrays are suitable for scenarios where the number of maps is fixed.