Go – Array of Strings
Arrays are a fixed-size collection of elements, and an array of strings is a collection where each element is of the type string
.
In this tutorial, we will cover the creation, retrieval, update, and deletion (CRUD) operations on string arrays, along with practical examples and explanations.
Syntax for Array of Strings
The syntax for declaring an array of strings is:
var arrayName [length]string
Here:
arrayName
: The name of the array.length
: The fixed size of the array (number of elements).string
: The type of elements in the array.
Basic CRUD Operations on Array of Strings
In the following examples, we will go through basic CRUD operations on Array of Strings, with detailed explanation for each program.
1 Create an Array of Strings
Here’s how to declare and initialize an array of strings:
package main
import "fmt"
func main() {
// Declare and initialize an array of strings
var fruits [3]string
fruits[0] = "Apple"
fruits[1] = "Banana"
fruits[2] = "Cherry"
fmt.Println("Array of Fruits:", fruits)
}
Explanation
- Declare Array: The array
fruits
is declared with a fixed size of 3 and element typestring
. - Assign Values: Elements are assigned values using their indices.
- Print Array: The entire array is printed using
fmt.Println
.
Output
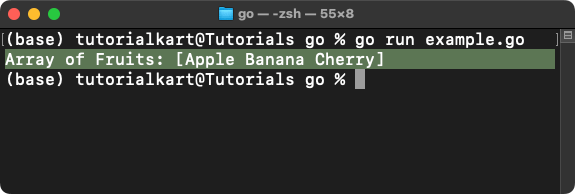
2 Retrieve Elements from Array
You can access array elements using their indices:
package main
import "fmt"
func main() {
// Declare and initialize an array of strings
fruits := [3]string{"Apple", "Banana", "Cherry"}
// Access and print individual elements
fmt.Println("First Fruit:", fruits[0])
fmt.Println("Second Fruit:", fruits[1])
fmt.Println("Third Fruit:", fruits[2])
}
Explanation
- Access Elements: Array elements are accessed using zero-based indexing.
- Print Elements: Individual elements are printed using their indices.
Output
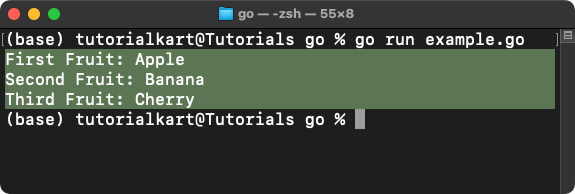
3 Update Elements in Array
Updating an array element is straightforward:
package main
import "fmt"
func main() {
// Declare and initialize an array of strings
fruits := [3]string{"Apple", "Banana", "Cherry"}
// Update an element
fruits[1] = "Blueberry"
// Print updated array
fmt.Println("Updated Array:", fruits)
}
Explanation
- Update Element: The second element of the array is updated using its index.
- Print Updated Array: The modified array is printed.
Output
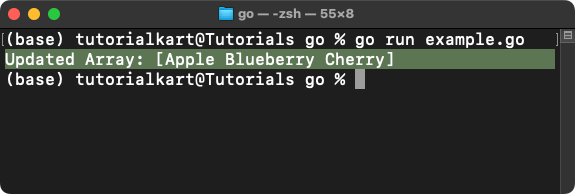
4 Delete an Element from Array
Since arrays in Go are of fixed size, you cannot delete elements directly. However, you can set the value to an empty string or use slices for dynamic behavior:
package main
import "fmt"
func main() {
// Declare and initialize an array of strings
fruits := [3]string{"Apple", "Banana", "Cherry"}
// "Delete" the second element by setting it to an empty string
fruits[1] = ""
// Print updated array
fmt.Println("Array after deletion:", fruits)
}
Explanation
- Set Empty Value: The second element is set to an empty string to simulate deletion.
- Print Array: The array with the updated value is printed.
Output
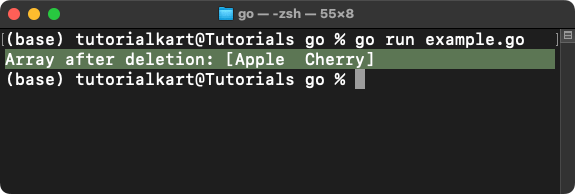
Points to Remember
- Fixed Length: Arrays in Go have a fixed size, and their length cannot be changed.
- Zero-Based Indexing: Array elements are accessed using indices starting from
0
. - Zero Value: Uninitialized string elements default to an empty string (
""
). - For Dynamic Behavior: Use slices instead of arrays when you need dynamic resizing.