Go – Array of Structs
Arrays in Go are fixed-size collections of elements of the same type. An array of structs is a collection where each element is a struct, allowing you to group and store multiple related fields for each element in a structured way.
In this tutorial, we will cover how to create and perform basic CRUD (Create, Read, Update, Delete) operations on arrays of structs with practical examples and detailed explanations.
Syntax for Array of Structs
The syntax to declare an array of structs involves defining a struct and using it as the element type:
type StructName struct {
Field1 dataType
Field2 dataType
...
}
var arrayName [length]StructName
Here:
StructName
: The name of the struct type used for elements.arrayName
: The name of the array.length
: The fixed size of the array.
Basic CRUD Operations on Struct Arrays
1 Create an Array of Structs
Here’s how to create and initialize an array of structs:
package main
import "fmt"
// Define a struct
type Employee struct {
Name string
ID int
Salary float64
}
func main() {
// Declare and initialize an array of Employee structs
employees := [2]Employee{
{"Alice", 101, 50000.0},
{"Bob", 102, 55000.0},
}
// Print the array
fmt.Println("Array of Employees:", employees)
}
Explanation
- Define Struct: The struct
Employee
is defined with fieldsName
,ID
, andSalary
. - Declare and Initialize: The array
employees
is initialized with twoEmployee
objects. - Print Array: The entire array is printed using
fmt.Println
.
Output
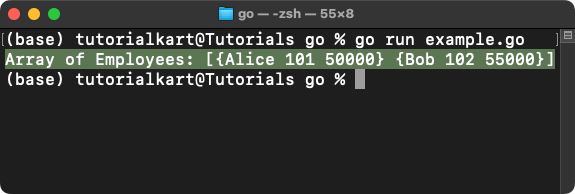
2 Read Elements from an Array
Access array elements using their indices and print individual fields:
package main
import "fmt"
// Define a struct
type Employee struct {
Name string
ID int
Salary float64
}
func main() {
// Declare and initialize an array of Employee structs
employees := [2]Employee{
{"Alice", 101, 50000.0},
{"Bob", 102, 55000.0},
}
// Access and print individual elements
fmt.Println("First Employee:", employees[0])
fmt.Println("Second Employee Name:", employees[1].Name)
}
Explanation
- Access Elements: Array elements are accessed using indices, and fields are accessed using the dot (
.
) operator. - Print Elements: Specific elements or fields are printed using
fmt.Println
.
Output
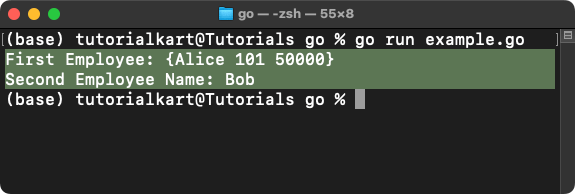
3 Update Elements in an Array
Update the fields of an element in the array:
package main
import "fmt"
// Define a struct
type Employee struct {
Name string
ID int
Salary float64
}
func main() {
// Declare and initialize an array of Employee structs
employees := [2]Employee{
{"Alice", 101, 50000.0},
{"Bob", 102, 55000.0},
}
// Update an employee's salary
employees[1].Salary = 60000.0
// Print updated array
fmt.Println("Updated Array:", employees)
}
Explanation
- Update Field: The
Salary
field of the secondEmployee
object is updated to60000.0
. - Print Updated Array: The modified array is printed using
fmt.Println
.
Output
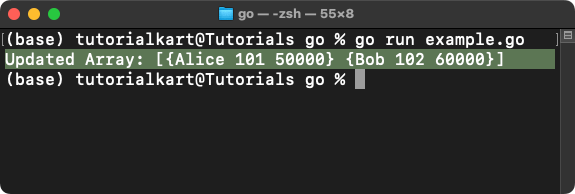
4 Delete Elements from an Array
To “delete” an object from the array, reset it to an empty struct:
package main
import "fmt"
// Define a struct
type Employee struct {
Name string
ID int
Salary float64
}
func main() {
// Declare and initialize an array of Employee structs
employees := [2]Employee{
{"Alice", 101, 50000.0},
{"Bob", 102, 55000.0},
}
// "Delete" the second employee by resetting it to an empty struct
employees[1] = Employee{}
// Print updated array
fmt.Println("Array after deletion:", employees)
}
Explanation
- Reset Element: The second element is reset to an empty
Employee
struct. - Print Updated Array: The array with the updated value is printed using
fmt.Println
.
Output
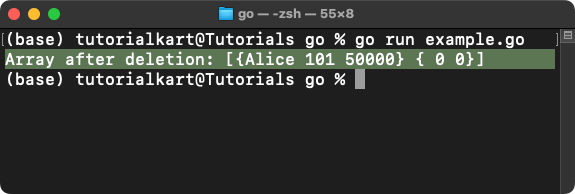
Points to Remember
- Fixed Size: Arrays in Go have a fixed size defined during declaration.
- Structured Data: Use structs to define the shape of objects stored in the array.
- Zero Value: Uninitialized elements in the array default to the zero value of the struct fields.
- Static Collections: Arrays are ideal for scenarios where the size and structure of the data are fixed.