Go – Array vs Slice
In Go, arrays and slices are both used to store collections of elements of the same type. However, they have significant differences in behavior and use cases. Arrays are fixed in size, while slices are dynamic and more flexible. Understanding the differences between arrays and slices is crucial for writing efficient and maintainable Go programs.
Differences Between Arrays and Slices
The following table summarizes the key differences between arrays and slices in Go:
Feature | Array | Slice |
---|---|---|
Size | Fixed at declaration | Dynamic, can grow and shrink |
Declaration | var arr [3]int | var s []int |
Resizing | Cannot be resized | Can be resized dynamically |
Memory Allocation | Allocated at compile-time | Allocated at runtime |
Default Value | Zero values for all elements | nil if not initialized |
Performance | Fast, as the size is fixed | Slightly slower due to dynamic resizing |
Use Case | Static collections | Dynamic collections |
Examples of Arrays and Slices
1. Example of an Array
Here’s an example of declaring, initializing, and using an array:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
var arr [3]int = [3]int{1, 2, 3}
// Print the array
fmt.Println("Array:", arr)
// Access elements by index
fmt.Println("First Element:", arr[0])
// Update an element
arr[1] = 10
fmt.Println("Updated Array:", arr)
}
Explanation
- Declare and Initialize: The array
arr
is declared with a fixed size of 3 and initialized with values{1, 2, 3}
. - Access Elements: Elements are accessed using zero-based indexing.
- Update Element: The value of the second element is updated to
10
.
Output
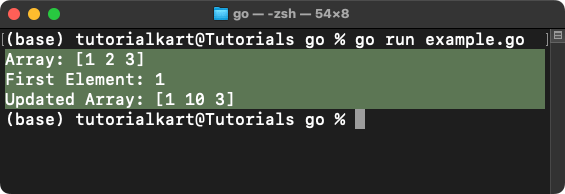
2. Example of a Slice
Here’s an example of declaring, initializing, and using a slice:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
var s []int = []int{1, 2, 3}
// Print the slice
fmt.Println("Slice:", s)
// Append an element
s = append(s, 4)
fmt.Println("Slice after append:", s)
// Access and update elements
s[1] = 10
fmt.Println("Updated Slice:", s)
}
Explanation
- Declare and Initialize: The slice
s
is declared and initialized with values{1, 2, 3}
. - Dynamic Resizing: The
append
function is used to add an element to the slice. - Access and Update: Elements can be accessed and updated similarly to arrays.
Output
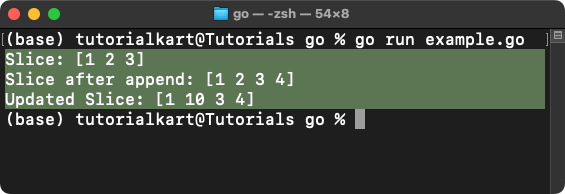
Points to Remember
- Use Arrays: When the size of the collection is fixed and known at compile-time.
- Use Slices: When the size of the collection needs to change dynamically at runtime.
- Pass by Value: Arrays are passed by value to functions, while slices are passed by reference.
- Flexibility: Slices provide more flexibility and are widely used in Go programming.