Go – Average of Two Numbers
In this tutorial, we will learn how to calculate the average of two numbers in the Go programming language (Golang). We’ll explore two methods: using variables directly and implementing a function for reusability. Each example will include the syntax, a program, and a detailed explanation to ensure a clear understanding of the concepts.
Syntax to Find Average of Two Numbers
The basic syntax for calculating the average of two numbers in Go is:
var average = (num1 + num2) / 2
Here, num1
and num2
are the numbers, and average
holds the result of their average calculation.
Note that we divide the sum of the two numbers by 2 to find the average.
Example 1: Average of Two Numbers Using Variables
In this example, we will define two integer variables, calculate their sum, divide it by 2 to find the average, and print the result to the console.
Program – example.go
package main
import "fmt"
func main() {
// Declare two variables
num1 := 10
num2 := 20
// Calculate the average
average := (num1 + num2) / 2
// Print the result
fmt.Println("The average of", num1, "and", num2, "is", average)
}
Explanation of Program
1. We use the :=
syntax to declare and initialize two integer variables, num1
and num2
, with values 10 and 20, respectively.
2. The expression (num1 + num2) / 2
calculates the average of the two variables by first adding them and then dividing by 2.
3. The result is stored in the average
variable.
4. The fmt.Println
function is used to print the result to the console, along with descriptive text.
Output
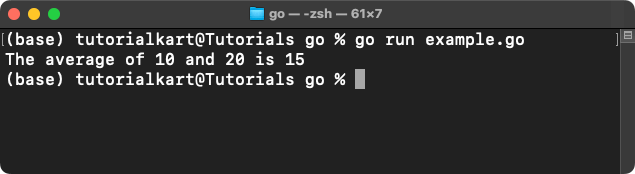
Example 2: Average of Two Numbers Using a Function
In this example, we will write a function to calculate the average of two numbers. This approach makes the code reusable, as the function can be called multiple times with different inputs.
Program – example.go
package main
import "fmt"
// Function to find the average of two numbers
func findAverage(a int, b int) int {
return (a + b) / 2
}
func main() {
// Call the function with two numbers
num1 := 15
num2 := 25
average := findAverage(num1, num2)
// Print the result
fmt.Println("The average of", num1, "and", num2, "is", average)
}
Explanation of Program
1. A function findAverage
is defined, which takes two integer parameters a
and b
, calculates their sum, and divides it by 2 to find the average.
2. Inside the main
function, two integers, num1
and num2
, are initialized with values 15 and 25.
3. The findAverage
function is called with num1
and num2
as arguments, and the result is stored in the average
variable.
4. The fmt.Println
function is used to print the result to the console, along with descriptive text.
Output
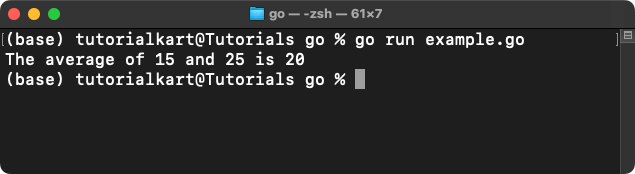