Go – Convert Array to Slice
In Go, arrays and slices are closely related data structures. While arrays have a fixed size, slices are dynamic and can grow or shrink. You can easily convert an array into a slice, enabling you to leverage the dynamic features of slices while working with the data stored in arrays.
In this tutorial, we will explore how to convert arrays into slices using simple syntax, along with practical examples and detailed explanations.
Syntax for Converting Array to Slice
The syntax to convert an array to a slice is straightforward:
slice := array[start:end]
Here:
array
: The array to be converted.start
: The starting index (inclusive).end
: The ending index (exclusive).
If you omit start
or end
, the slice includes all elements from the beginning or until the end of the array, respectively.
Examples of Converting Array to Slice
1. Convert Entire Array to Slice
Here’s how to convert an entire array into a slice:
package main
import "fmt"
func main() {
// Declare and initialize an array
var arr = [5]int{10, 20, 30, 40, 50}
// Convert the entire array to a slice
slice := arr[:]
// Print the slice
fmt.Println("Slice:", slice)
}
Explanation
- Declare Array: The array
arr
is declared and initialized with 5 elements. - Convert to Slice: The entire array is converted into a slice using the slice expression
arr[:]
. - Print Slice: The resulting slice is printed using
fmt.Println
.
Output
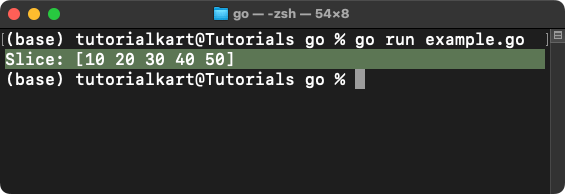
2. Convert Partial Array to Slice
Here’s how to convert a portion of an array into a slice:
package main
import "fmt"
func main() {
// Declare and initialize an array
var arr = [5]int{10, 20, 30, 40, 50}
// Convert part of the array to a slice
slice := arr[1:4]
// Print the slice
fmt.Println("Slice:", slice)
}
Explanation
- Declare Array: The array
arr
is declared and initialized with 5 elements. - Convert to Slice: A portion of the array (from index 1 to index 3) is converted into a slice using
arr[1:4]
. - Print Slice: The resulting slice is printed using
fmt.Println
.
Output
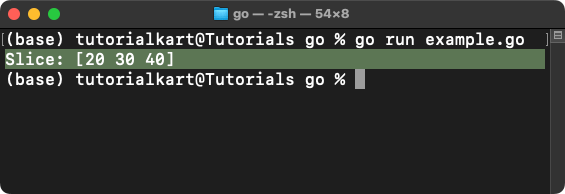
3. Convert Array to Slice and Modify Elements
When you convert an array to a slice, changes to the slice affect the original array:
package main
import "fmt"
func main() {
// Declare and initialize an array
var arr = [5]int{10, 20, 30, 40, 50}
// Convert the array to a slice
slice := arr[:]
// Modify an element in the slice
slice[2] = 99
// Print the updated slice and array
fmt.Println("Slice:", slice)
fmt.Println("Array:", arr)
}
Explanation
- Convert to Slice: The entire array is converted into a slice using
arr[:]
. - Modify Slice: An element in the slice is updated using its index.
- Reflect Changes: The changes to the slice are reflected in the original array since slices share the underlying array memory.
Output
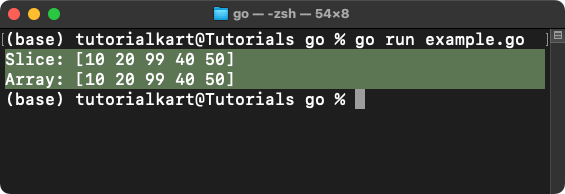
Points to Remember
- Shared Memory: Slices share the same underlying memory as the array. Modifications to the slice will affect the original array.
- Partial Conversion: You can convert a portion of an array into a slice using the slice expression
[start:end]
. - Dynamic Nature: Once converted, slices can be resized dynamically using functions like
append
. - Default Behavior: Omitting
start
orend
in the slice expression includes all elements from the start or until the end, respectively.