Go – Convert Slice to Array
In Go, slices are dynamic, while arrays have a fixed size. Converting a slice to an array requires that the slice’s length matches the size of the target array. This operation is useful when you need to work with fixed-size collections for better memory management or compatibility with functions that expect arrays.
In this tutorial, we will explore how to convert slices to arrays in Go with practical examples and detailed explanations.
Key Points About Slice to Array Conversion
- Size Matching: The slice’s length must match the size of the target array.
- Explicit Conversion: Use explicit conversion to copy slice elements into an array.
- Performance: Arrays provide better performance for fixed-size collections since they avoid dynamic resizing.
Examples of Converting Slice to Array
1 Convert Slice to Array Using Explicit Copy
This example demonstrates how to convert a slice to an array by explicitly copying elements:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
slice := []int{1, 2, 3, 4, 5}
// Create an array with the same length as the slice
var array [5]int
// Copy elements from the slice to the array
for i, v := range slice {
array[i] = v
}
// Print the array
fmt.Println("Array:", array)
}
Explanation
- Declare Slice: The slice
slice
is initialized with values{1, 2, 3, 4, 5}
. - Create Array: An array
array
of size 5 is declared to match the length of the slice. - Copy Elements: A
for
loop iterates through the slice, copying each element to the corresponding index in the array. - Print Result: The array is printed to verify the conversion.
Output
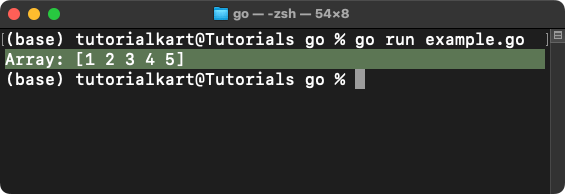
2 Convert Slice to Array Using Array Literal
If the slice’s length and the array’s size are known, you can directly assign slice elements to an array literal:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
slice := []int{10, 20, 30, 40, 50}
// Convert the slice to an array using an array literal
array := [5]int{slice[0], slice[1], slice[2], slice[3], slice[4]}
// Print the array
fmt.Println("Array:", array)
}
Explanation
- Declare Slice: The slice
slice
is initialized with values{10, 20, 30, 40, 50}
. - Create Array Literal: An array
array
is initialized using the slice’s elements explicitly. - Print Result: The array is printed to confirm the conversion.
Output
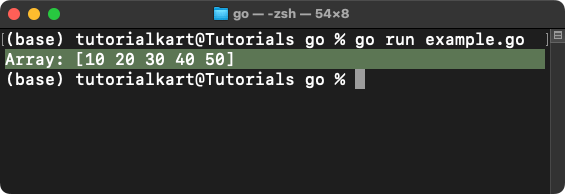
Points to Remember
- Size Matching: The size of the array must match the length of the slice for successful conversion.
- Explicit Copy: Use a
for
loop or array literal for copying slice elements to an array. - Immutability: Once converted, the array is independent and cannot dynamically grow like a slice.
- Use Case: Converting slices to arrays is useful when working with functions that require fixed-size arrays.