Go – Convert Slice to Map
In Go, slices are used for dynamic collections of elements, while maps are key-value pairs that offer fast lookups. Converting a slice to a map involves mapping each element of the slice to a key in the map, based on a specific logic or transformation.
In this tutorial, we will explore how to convert slices to maps in Go using practical examples and detailed explanations.
Steps to Convert Slice to Map
- Initialize a Map: Create an empty map to store the key-value pairs.
- Iterate Through Slice: Use a
for
loop to iterate through the slice. - Assign Keys and Values: Define the logic for mapping slice elements to keys and values in the map.
Examples of Converting Slice to Map
1. Convert Slice to Map Using Index as Key
This example demonstrates how to convert a slice to a map using the slice index as the map key:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
slice := []string{"apple", "banana", "cherry"}
// Create an empty map
result := make(map[int]string)
// Convert slice to map
for i, v := range slice {
result[i] = v
}
// Print the resulting map
fmt.Println("Map:", result)
}
Explanation
- Declare Slice: The slice
slice
is initialized with values{"apple", "banana", "cherry"}
. - Create Map: An empty map
result
is created with integer keys and string values. - Assign Key-Value Pairs: A
for
loop iterates through the slice, mapping each index to its corresponding value in the map. - Print Result: The resulting map is printed using
fmt.Println
.
Output
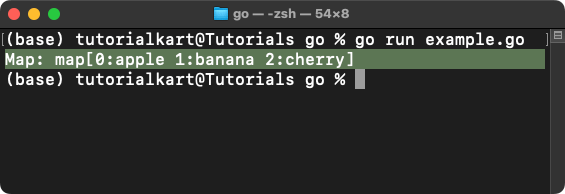
2. Convert Slice to Map Using Element as Key
This example demonstrates how to convert a slice to a map using the slice element as the map key:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
slice := []string{"apple", "banana", "cherry"}
// Create an empty map
result := make(map[string]int)
// Convert slice to map
for i, v := range slice {
result[v] = i
}
// Print the resulting map
fmt.Println("Map:", result)
}
Explanation
- Declare Slice: The slice
slice
is initialized with values{"apple", "banana", "cherry"}
. - Create Map: An empty map
result
is created with string keys and integer values. - Assign Key-Value Pairs: A
for
loop iterates through the slice, mapping each element to its index in the map. - Print Result: The resulting map is printed using
fmt.Println
.
Output
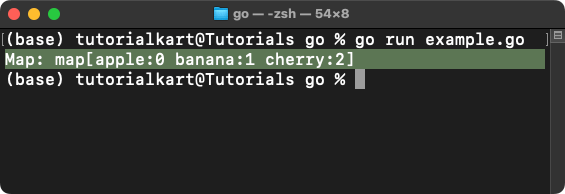
3. Convert Slice to Map Using Custom Logic
This example demonstrates how to apply custom logic when converting a slice to a map:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize a slice
slice := []int{2, 4, 6, 8}
// Create an empty map
result := make(map[int]int)
// Convert slice to map
for _, v := range slice {
result[v] = v * v
}
// Print the resulting map
fmt.Println("Map:", result)
}
Explanation
- Declare Slice: The slice
slice
is initialized with values{2, 4, 6, 8}
. - Create Map: An empty map
result
is created with integer keys and integer values. - Custom Logic: Each element is mapped to its square as the value in the map.
- Print Result: The resulting map is printed using
fmt.Println
.
Output
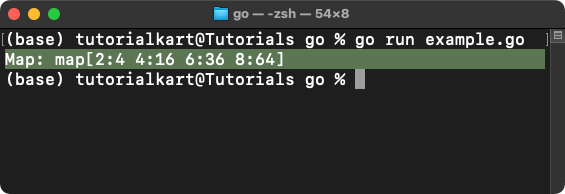
Points to Remember
- Logic-Driven Keys and Values: Define clear logic for mapping slice elements to keys and values in the map.
- Map Initialization: Always initialize the map using
make
before populating it. - Dynamic Mapping: You can map slice elements to any type of key-value pairs based on your requirements.
- Memory Independence: The map is independent of the original slice after conversion.