Go – Convert Slice to String
In Go, slices are dynamic data structures used to store collections of elements, while strings are immutable sequences of characters. Converting a slice to a string is a common requirement when working with text data or preparing output for display or storage. This conversion can be performed in different ways depending on the type of elements in the slice.
In this tutorial, we will explore how to convert slices to strings in Go using practical examples and detailed explanations.
Key Considerations for Slice to String Conversion
- Element Type: The conversion method depends on the type of elements in the slice (e.g.,
byte
,rune
, orstring
). - Concatenation: For slices of strings, use
strings.Join
for efficient concatenation. - Encoding: For slices of bytes, convert them directly to a string using
string(slice)
.
Examples of Converting Slice to String
1 Convert Slice of Bytes to String
This example demonstrates how to convert a slice of bytes ([]byte
) to a string:
package main
import "fmt"
func main() {
// Declare and initialize a slice of bytes
byteSlice := []byte{'H', 'e', 'l', 'l', 'o'}
// Convert the slice of bytes to a string
str := string(byteSlice)
// Print the resulting string
fmt.Println("String:", str)
}
Explanation
- Declare Byte Slice: The slice
byteSlice
is initialized with byte values representing the characters'H'
,'e'
,'l'
,'l'
, and'o'
. - Convert to String: The
string
function is used to convert the byte slice into a string. - Print Result: The resulting string
"Hello"
is printed usingfmt.Println
.
Output
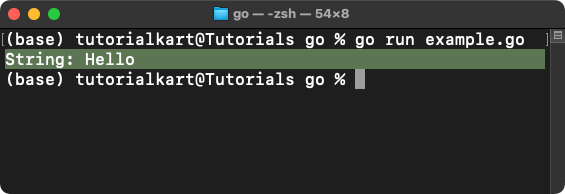
2 Convert Slice of Strings to Single String
This example demonstrates how to convert a slice of strings ([]string
) to a single string using strings.Join
:
package main
import (
"fmt"
"strings"
)
func main() {
// Declare and initialize a slice of strings
stringSlice := []string{"Go", "is", "awesome"}
// Convert the slice of strings to a single string
str := strings.Join(stringSlice, " ")
// Print the resulting string
fmt.Println("String:", str)
}
Explanation
- Declare String Slice: The slice
stringSlice
is initialized with values{"Go", "is", "awesome"}
. - Join Strings: The
strings.Join
function concatenates the elements of the slice into a single string, separated by a space (" "
). - Print Result: The resulting string
"Go is awesome"
is printed usingfmt.Println
.
Output
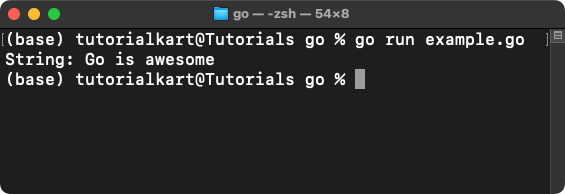
3 Convert Slice of Runes to String
This example demonstrates how to convert a slice of runes ([]rune
) to a string:
package main
import "fmt"
func main() {
// Declare and initialize a slice of runes
runeSlice := []rune{'世', '界', '你', '好'}
// Convert the slice of runes to a string
str := string(runeSlice)
// Print the resulting string
fmt.Println("String:", str)
}
Explanation
- Declare Rune Slice: The slice
runeSlice
is initialized with Unicode characters'世'
,'界'
,'你'
, and'好'
. - Convert to String: The
string
function converts the rune slice into a string. - Print Result: The resulting string
"世界你好"
is printed usingfmt.Println
.
Output
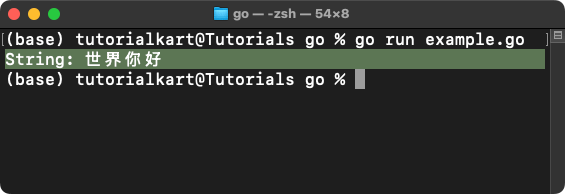
Points to Remember
- Type-Specific Conversion: Use
string(slice)
for slices ofbyte
orrune
, andstrings.Join
for slices of strings. - Efficient Concatenation: The
strings.Join
function is more efficient than manually concatenating strings in a loop. - Immutability: Strings in Go are immutable, so any modifications require creating a new string.
- Encoding: Ensure that the elements in your slice (e.g., bytes or runes) are encoded correctly for meaningful string output.