Go – Count Digits in Number
In this tutorial, we will learn how to count the number of digits in a given number using the Go programming language (Golang). Counting digits is a fundamental task in programming that demonstrates the use of loops and arithmetic operations. We will provide a detailed explanation and example program to accomplish this task.
Logic to Count Digits in a Number
The logic for counting digits in a number involves repeatedly dividing the number by 10 and counting the iterations:
- Initialize Counter: Start with a counter variable set to 0 to keep track of the number of digits.
- Divide by 10: Use integer division (
/
) to remove the last digit of the number. - Increment Counter: Increment the counter with each iteration.
- Repeat: Continue dividing the number by 10 until it becomes 0.
- Handle Special Case: If the number is 0, it has 1 digit.
Example Program to Count Digits in a Number
Program – example.go
</>
Copy
package main
import "fmt"
func main() {
// Declare a variable to hold the input number
var num int
// Prompt the user to enter a number
fmt.Print("Enter an integer: ")
fmt.Scan(&num)
// Handle negative numbers by converting them to positive
if num < 0 {
num = -num
}
// Special case for 0
if num == 0 {
fmt.Println("The number 0 has 1 digit.")
return
}
// Initialize a counter for the digits
count := 0
// Count the digits
for num != 0 {
num /= 10
count++
}
// Display the number of digits
fmt.Printf("The number has %d digits.\n", count)
}
Explanation of Program
- Declare a Variable: An integer variable,
num
, is declared to store the user input. - Prompt the User: The program prompts the user to enter an integer using the
fmt.Print
function. - Handle Negative Numbers: If the number is negative, it is converted to a positive number using
-num
to ensure correctness. - Handle Special Case for 0: If the input is 0, the program directly prints that the number has 1 digit and exits.
- Count the Digits: A loop is used to repeatedly divide the number by 10 until it becomes 0. A counter variable
count
is incremented in each iteration to track the number of digits. - Display the Result: The program prints the total count of digits using
fmt.Printf
.
Output
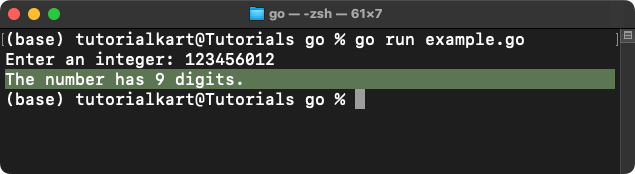
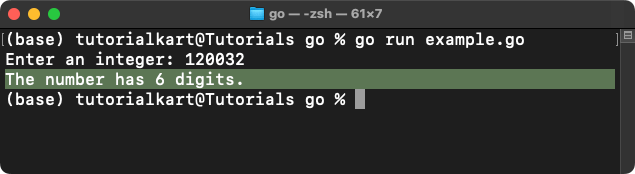
This program efficiently counts the digits in an integer, including special cases such as 0 and negative numbers.