Go – Create Array of Specific Length
Arrays in Go are collections of elements with the same type, and their length is fixed and defined at the time of creation. In this tutorial, we will explore the syntax, examples, and best practices for creating and initializing arrays with specific lengths.
Syntax for Creating an Array
The syntax to create an array of a specific length is:
</>
Copy
var arrayName [length]elementType
Here:
arrayName
: The name of the array.length
: The number of elements the array can hold (fixed size).elementType
: The type of elements in the array (e.g.,int
,float64
,string
, etc.).
Examples of Creating Arrays
Example 1: Create an Array of Integers
Here’s an example of creating an array of integers with a specific length:
</>
Copy
package main
import "fmt"
func main() {
// Declare an array of 5 integers
var numbers [5]int
// Assign values to the array
numbers[0] = 10
numbers[1] = 20
numbers[2] = 30
numbers[3] = 40
numbers[4] = 50
// Print the array
fmt.Println("Array:", numbers)
}
Explanation
- Declare the Array: The array
numbers
is declared with a fixed length of 5 and element typeint
. - Assign Values: Individual elements are assigned values using their indices.
- Print the Array: The entire array is printed using
fmt.Println
.
Output
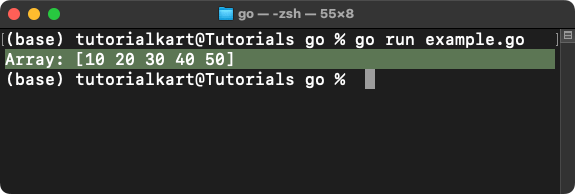
Example 2: Initialize an Array with Values
Arrays can also be initialized with values at the time of declaration:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array
numbers := [5]int{10, 20, 30, 40, 50}
// Print the array
fmt.Println("Array:", numbers)
}
Explanation
- Declare and Initialize: The array
numbers
is declared with a fixed length of 5 and initialized with values{10, 20, 30, 40, 50}
. - Print the Array: The entire array is printed using
fmt.Println
.
Output
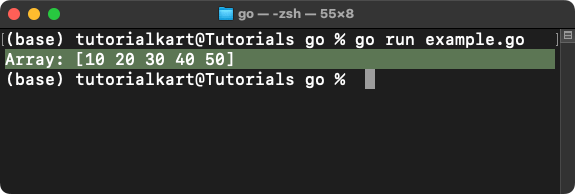
Example 3: Create an Array of Strings
Here’s an example of creating an array of strings:
</>
Copy
package main
import "fmt"
func main() {
// Declare and initialize an array of strings
fruits := [3]string{"Apple", "Banana", "Cherry"}
// Print the array
fmt.Println("Array:", fruits)
}
Explanation
- Declare and Initialize: The array
fruits
is declared with a fixed length of 3 and initialized with string values{"Apple", "Banana", "Cherry"}
. - Print the Array: The entire array is printed using
fmt.Println
.
Output
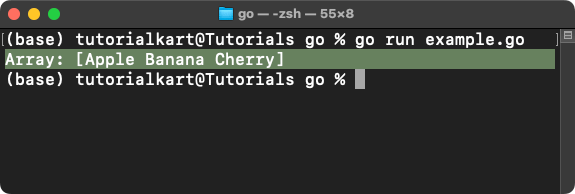
Example 4: Create an Array Using Default Values
If no values are assigned to the array, its elements are initialized with the zero value of the element type:
</>
Copy
package main
import "fmt"
func main() {
// Declare an array of 4 floats
var scores [4]float64
// Print the array
fmt.Println("Array with default values:", scores)
}
Explanation
- Declare the Array: The array
scores
is declared with a fixed length of 4 and element typefloat64
. - Default Values: Since no values are assigned, all elements are initialized to the zero value for
float64
, which is0.0
. - Print the Array: The entire array is printed using
fmt.Println
.
Output
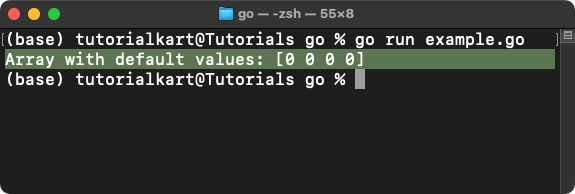
Points to Remember
- Fixed Length: The size of an array in Go is fixed and cannot be changed after declaration.
- Zero-Based Indexing: Array elements are accessed using indices starting from
0
. - Zero Value Initialization: Uninitialized elements in an array default to the zero value of the element type.