Go – Even or Odd Number
In this tutorial, we will learn how to determine whether a number is even or odd using the Go programming language (Golang). Identifying whether a number is even or odd is a task that demonstrates the use of conditional logic and the modulus operator. We will provide a simple program with detailed explanations to implement this functionality.
Logic to Check Even or Odd
A number is classified as even or odd based on its divisibility by 2:
- Even Number: If a number is divisible by 2 (i.e., the remainder when divided by 2 is 0), it is an even number.
- Odd Number: If a number is not divisible by 2 (i.e., the remainder when divided by 2 is not 0), it is an odd number.
This logic can be implemented using the modulus operator (%
) in Go.
Example Program to Check Even or Odd
In this example, we will read an integer from user, and use modulus operator to find if the input integer is an even or odd number.
Program – example.go
</>
Copy
package main
import "fmt"
func main() {
// Declare a variable to hold the number
var num int
// Prompt the user to enter a number
fmt.Print("Enter an integer: ")
fmt.Scan(&num)
// Check if the number is even or odd
if num%2 == 0 {
fmt.Println(num, "is an even number.")
} else {
fmt.Println(num, "is an odd number.")
}
}
Explanation of Program
- Declare a Variable: An integer variable,
num
, is declared to store the user input. - Prompt the User: The program prompts the user to enter an integer using the
fmt.Print
function. - Read the Input: The
fmt.Scan
function reads the input and stores it in the variablenum
. - Check Even or Odd: The modulus operator (
%
) is used to check if the number is divisible by 2. If the remainder is 0, the number is even; otherwise, it is odd. We used if else statement for this. - Print the Result: The program prints whether the number is even or odd based on the condition.
Output
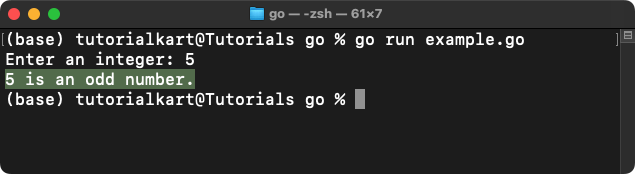
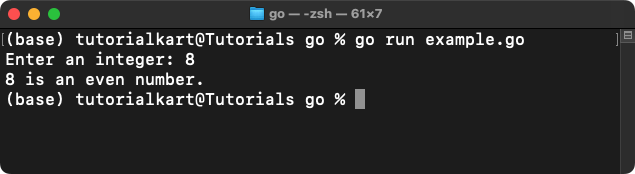
This program efficiently determines whether a number is even or odd by leveraging the modulus operator and conditional statements.