Go – For Loop Array
Arrays are fixed-length sequences of elements of the same type. Using a For Loop, you can efficiently process each element of an array.
In this tutorial, we will provide practical examples and explanations to demonstrate how to iterate over arrays using For loop.
Iterating Over Arrays Using For Loop
You can use a for
loop to iterate over an array either by index or using the range
keyword. Below are examples of both approaches.
Example 1: Iterating Over an Array Using Index
In this example, we will use a standard for
loop to iterate over an array using its indices:
package main
import "fmt"
func main() {
// Declare an array
arr := [5]int{10, 20, 30, 40, 50}
// Iterate over the array using a for loop
for i := 0; i < len(arr); i++ {
fmt.Printf("Index: %d, Value: %d\n", i, arr[i])
}
}
Explanation
- Declare an Array: The array
arr
of size 5 is initialized with integers. - Set up the For Loop: The
for
loop starts at index 0 and runs until it reaches the length of the array usinglen(arr)
. - Access Elements: Inside the loop,
arr[i]
accesses the value of the array at the current indexi
. - Print Elements: The index and value of each element are printed using
fmt.Printf
.
Output
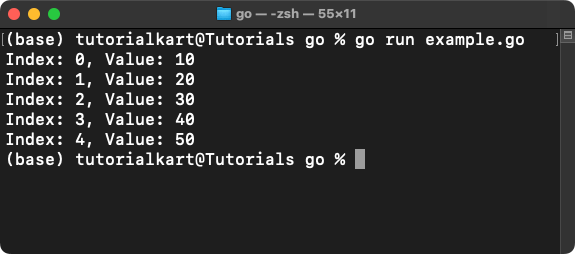
Example 2: Iterating Over an Array Using Range
Using the range
keyword simplifies iteration by providing both the index and value of each element:
package main
import "fmt"
func main() {
// Declare an array
arr := [5]int{10, 20, 30, 40, 50}
// Iterate over the array using range
for index, value := range arr {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}
Explanation
- Declare an Array: The array
arr
of size 5 is initialized with integers. - Use Range: The
range
keyword is used to iterate over the array, providing both the index and value. - Access Elements:
index
stores the position, andvalue
holds the element at that position. - Print Elements: The index and value are printed using
fmt.Printf
.
Output
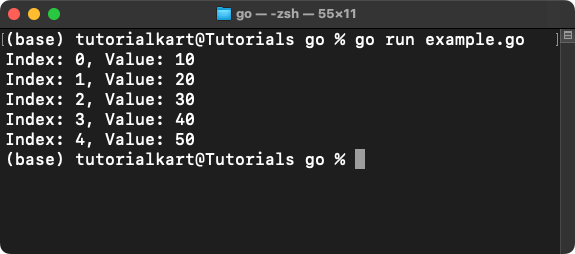
Example 3: Skipping Index or Value
In some cases, you may only need the index or the value. You can use the underscore (_
) to ignore the one you don’t need:
package main
import "fmt"
func main() {
// Declare an array
arr := [5]int{10, 20, 30, 40, 50}
// Only print values
for _, value := range arr {
fmt.Println("Value:", value)
}
// Only print indices
for index := range arr {
fmt.Println("Index:", index)
}
}
Explanation
- Ignore Index: Use
_
in place of the index when you only need the value. - Ignore Value: Use
_
in place of the value when you only need the index. - Iterate and Print: The loop iterates through the array, printing either the value or index as required.
Output
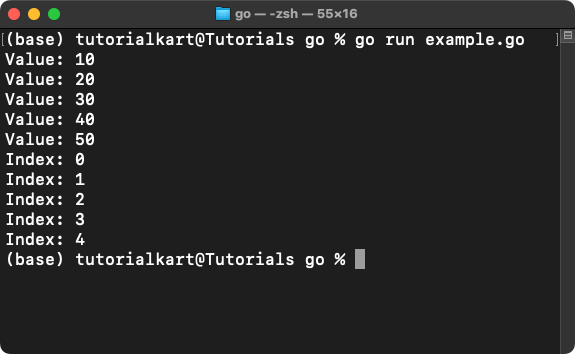
When to Use For Loop with Arrays
You can use For Loop with Arrays:
- To access each element of the array for processing or computation.
- To find specific elements based on conditions.
- To iterate over arrays for transformations or aggregations.
Conclusion
The for
loop in Go is a powerful tool for iterating over arrays. Using either index-based iteration or the range
keyword, you can efficiently access and manipulate array elements.