Go – For Loop Break
The break
statement is used to terminate a for loop prematurely when a specific condition is met. This is useful when you need to exit the loop without completing all iterations.
In this tutorial, we will discuss the syntax and provide examples to demonstrate how to use a break statement in a For Loop.
Syntax of Break in For Loop
The break
statement is used inside a loop to terminate its execution immediately:
for initialization; condition; post {
if conditionToBreak {
break
}
// Code to execute if conditionToBreak is false
}
Example 1: Breaking Out of a For Loop
Here’s an example of using break
to stop a loop when a specific condition is met:
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i == 5 {
fmt.Println("Breaking the loop at i =", i)
break
}
fmt.Println(i)
}
}
Output
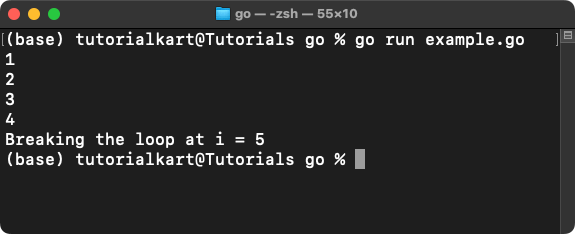
Example 2: Breaking Out of an Infinite Loop
The break
statement is commonly used to terminate infinite loops:
package main
import "fmt"
func main() {
count := 1
for {
fmt.Println("Iteration:", count)
if count == 5 {
fmt.Println("Breaking the infinite loop.")
break
}
count++
}
}
Output
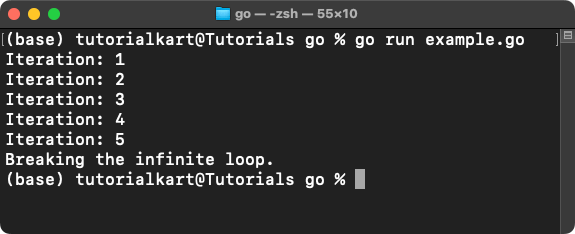
Example 3: Breaking Out of Nested Loops
In nested loops, break
will terminate only the innermost loop. To break out of all loops, you can use a labeled break:
package main
import "fmt"
func main() {
outer:
for i := 1; i <= 3; i++ {
for j := 1; j <= 3; j++ {
if i == 2 && j == 2 {
fmt.Println("Breaking out of nested loops.")
break outer // Exit both loops
}
fmt.Printf("i: %d, j: %d\n", i, j)
}
}
}
Output
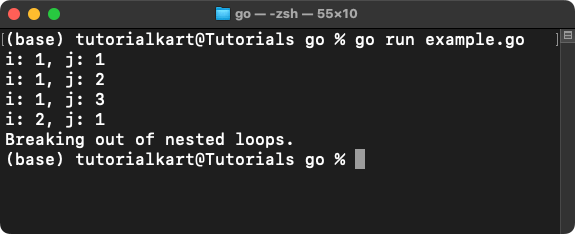
When to Use Break in a For Loop
You can use a break statement in a For loop:
- To terminate a loop early when a specific condition is met.
- To exit infinite loops when the desired condition occurs.
- To optimize performance by avoiding unnecessary iterations.
Conclusion
The break
statement in Go is used for controlling the execution of loops. By using break
, you can efficiently exit loops when necessary.