Go – For Loop Continue
The continue
statement is used to skip the remaining code in the current iteration of a loop and move to the next iteration. This is useful when you want to bypass certain iterations based on specific conditions.
In this tutorial, we will explore the syntax and practical examples to demonstrate the usage of continue
in a For Loop.
Syntax of Continue in For Loop
The continue
statement can be used inside a for
loop to skip the rest of the current iteration:
for initialization; condition; post {
if conditionToSkip {
continue
}
// Code to execute if conditionToSkip is false
}
Example 1: Skipping Even Numbers
Let’s create a program to print odd numbers from 1 to 10, skipping even numbers using continue
:
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i%2 == 0 {
continue // Skip even numbers
}
fmt.Println(i) // Print odd numbers
}
}
Output
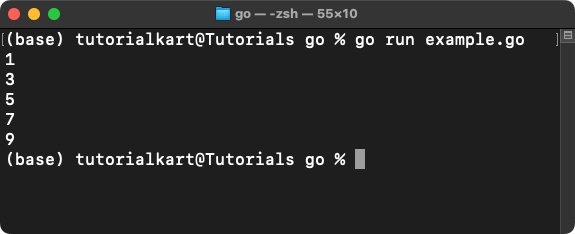
Example 2: Skipping Specific Iterations in For Loop
Here’s an example of skipping specific iterations, such as skipping multiples of 3:
package main
import "fmt"
func main() {
for i := 1; i <= 10; i++ {
if i%3 == 0 {
continue // Skip multiples of 3
}
fmt.Println(i)
}
}
Output
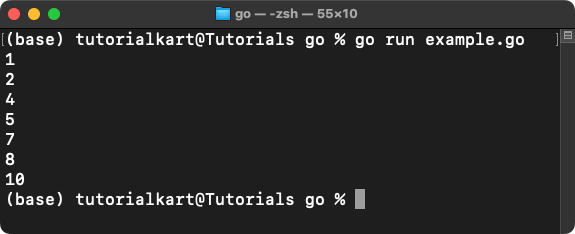
Example 3: Continue in Nested For Loop
The continue
statement can also be used in nested loops to skip iterations of the inner loop:
package main
import "fmt"
func main() {
for i := 1; i <= 3; i++ {
for j := 1; j <= 3; j++ {
if j == 2 {
continue // Skip the inner loop iteration when j == 2
}
fmt.Printf("i: %d, j: %d\n", i, j)
}
}
}
Output
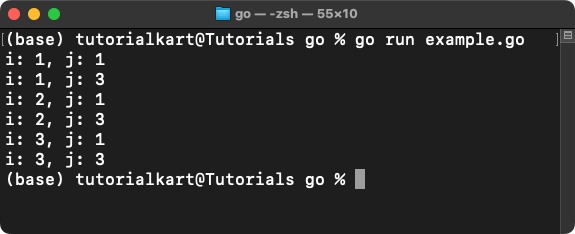
When to Use Continue in a For Loop
- To skip unnecessary iterations and improve efficiency.
- To handle specific cases differently without exiting the loop.
- To simplify logic by avoiding deeply nested conditional statements.
Conclusion
The continue
statement in Go allows you to skip specific iterations in a for
loop. It is a powerful tool to simplify logic and handle exceptions within loops effectively. Understanding its use in different scenarios will make your loops more flexible and efficient.