Go – For Loop Examples
In this tutorial, we will explore the examples for For Loop in the Go programming language (Golang). The for
loop is a control structure that allows code to be executed repeatedly based on a condition. Go simplifies looping with a single for
construct, making it powerful and versatile. We will cover basic syntax, variations of the for
loop, and practical examples.
Syntax of For Loop
The basic syntax of a for
loop in Go is:
for initialization; condition; post {
// Code to execute
}
Here:
- Initialization: Initializes the loop variable (executed once).
- Condition: The loop runs while this condition evaluates to
true
. - Post: Updates the loop variable after each iteration.
Example of a Basic For Loop
package main
import "fmt"
func main() {
// Basic for loop to print numbers from 1 to 5
for i := 1; i <= 5; i++ {
fmt.Println(i)
}
}
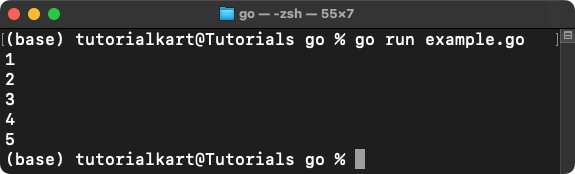
Variations of For Loop
1. Infinite Loop
A for
loop without initialization, condition, or post becomes an infinite loop:
package main
import "fmt"
func main() {
count := 0
for {
fmt.Println("Infinite loop")
count++
if count == 3 {
break // Exit the loop after 3 iterations
}
}
}
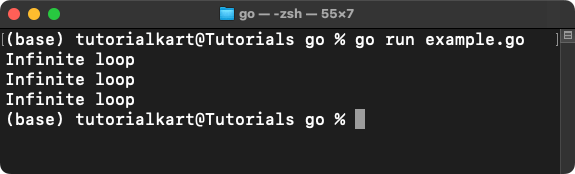
If there is no break statement, then the loop would have run indefinitely.
package main
import "fmt"
func main() {
count := 0
for {
fmt.Println("Infinite loop")
count++
}
}
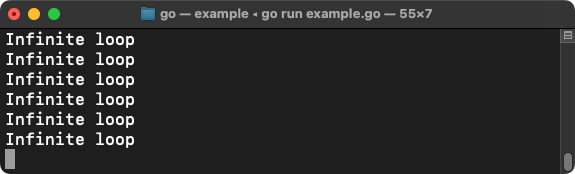
2. While-Like Loop
A for
loop can mimic a while
loop by omitting initialization and post statements:
package main
import "fmt"
func main() {
count := 1
for count <= 5 {
fmt.Println(count)
count++
}
}
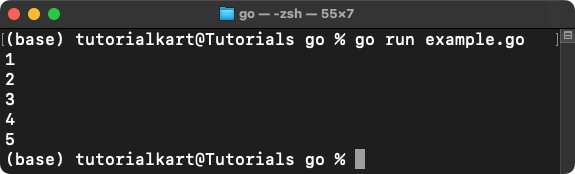
3. Loop Over Arrays or Slices
You can use a for
loop to iterate over arrays or slices:
package main
import "fmt"
func main() {
nums := []int{10, 20, 30, 40, 50}
for i := 0; i < len(nums); i++ {
fmt.Println("Index:", i, "Value:", nums[i])
}
}
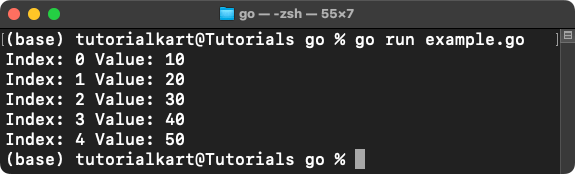
4. Range Loop
The range
keyword simplifies iterating over arrays, slices, maps, and strings:
package main
import "fmt"
func main() {
nums := []int{10, 20, 30, 40, 50}
for index, value := range nums {
fmt.Println("Index:", index, "Value:", value)
}
}
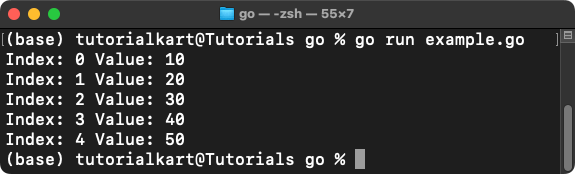
5. Nested Loops
A for
loop can be nested within another for
loop:
package main
import "fmt"
func main() {
for i := 1; i <= 3; i++ {
for j := 1; j <= 3; j++ {
fmt.Printf("i: %d, j: %d\n", i, j)
}
}
}
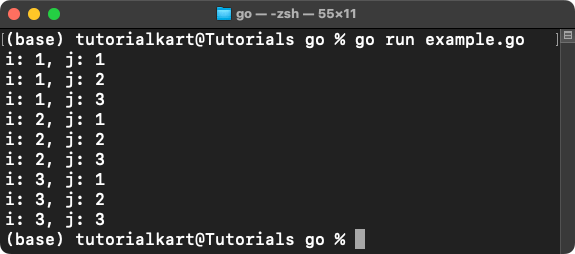
Break and Continue in For Loops
The break
statement exits the loop prematurely, while the continue
statement skips the current iteration and moves to the next:
package main
import "fmt"
func main() {
for i := 1; i <= 5; i++ {
if i == 3 {
continue // Skip iteration for i == 3
}
if i == 5 {
break // Exit the loop when i == 5
}
fmt.Println(i)
}
}
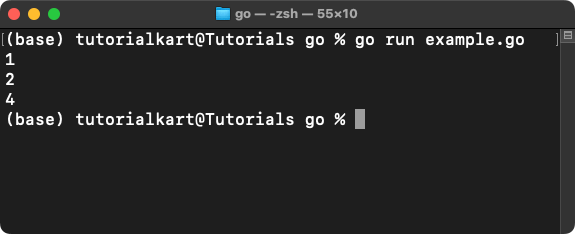
Conclusion
The for
loop in Go is versatile and powerful, allowing for various loop constructs such as infinite loops, while-like loops, and range-based iterations.