Go – For Loop Map
Maps in Go are key-value pairs, and the for
loop with the range
keyword is commonly used to traverse them.
In this tutorial, we will provide practical examples along with detailed explanations to demonstrate how to iterate over maps using For Loop.
Iterating Over Maps Using For Loop
The range
keyword allows you to iterate over maps in Go. It returns two values during each iteration: the key and the value.
Example 1: Iterating Over a Map
In this example, we will iterate over a map and print both its keys and values:
package main
import "fmt"
func main() {
// Declare a map
countries := map[string]string{
"US": "United States",
"IN": "India",
"JP": "Japan",
}
// Iterate over the map
for key, value := range countries {
fmt.Printf("Key: %s, Value: %s\n", key, value)
}
}
Explanation
- Declare a Map: A map named
countries
is initialized with key-value pairs where keys are country codes, and values are country names. - Use Range: The
range
keyword iterates over the map, returning each key and its corresponding value. - Print Key-Value Pairs: The key and value are printed using
fmt.Printf
.
Output
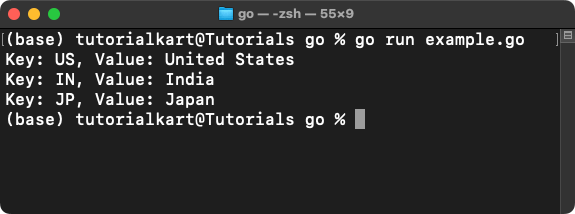
Example 2: Iterating Over Keys Only
If you only need the keys of a map, you can ignore the value by using the underscore (_
):
package main
import "fmt"
func main() {
// Declare a map
countries := map[string]string{
"US": "United States",
"IN": "India",
"JP": "Japan",
}
// Iterate over the map keys
for key := range countries {
fmt.Println("Key:", key)
}
}
Explanation
- Declare a Map: The map
countries
is initialized with key-value pairs. - Use Range: The
range
keyword is used to iterate over the map keys. - Ignore Values: By omitting the value and using only the key, the loop focuses on printing the keys.
Output
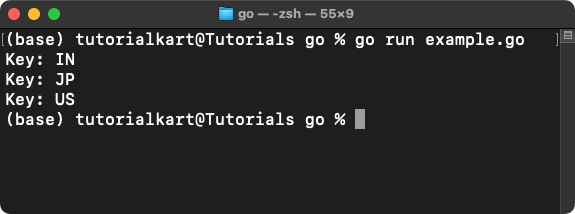
Example 3: Iterating Over Values Only
If you only need the values of a map, you can ignore the keys:
package main
import "fmt"
func main() {
// Declare a map
countries := map[string]string{
"US": "United States",
"IN": "India",
"JP": "Japan",
}
// Iterate over the map values
for _, value := range countries {
fmt.Println("Value:", value)
}
}
Explanation
- Declare a Map: The map
countries
is initialized with key-value pairs. - Use Range: The
range
keyword iterates over the map, returning only the values. - Ignore Keys: The underscore (
_
) is used to ignore the keys while focusing on the values.
Output
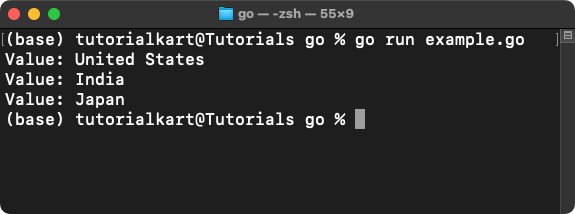
When to Use For Loop with Maps
You can use For Loop with Maps:
- To process or manipulate both keys and values.
- To retrieve all keys or values for specific operations.
- To filter or search for specific elements in the map.
Conclusion
The for
loop with range
provides a simple and efficient way to iterate over maps in Go. By selectively using keys or values, you can handle map data effectively to suit your program’s requirements. Understanding these patterns will help you process and manipulate maps efficiently.