Go – For Loop Range
The range
keyword is used with the for
loop to iterate over elements of arrays, slices, maps, strings, or channels. It simplifies iteration by providing both the index and the value of elements.
In this tutorial, we will cover its syntax and usage with practical examples.
Syntax of For Loop with Range
The syntax of the for
loop with range
is:
for index, value := range collection {
// Code to execute for each element
}
Here:
- index: The index of the current element.
- value: The value of the current element.
- collection: The array, slice, map, string, or channel being iterated.
Example 1: Iterating Over a Slice
Let’s iterate over a slice of integers:
package main
import "fmt"
func main() {
numbers := []int{10, 20, 30, 40, 50}
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}
Output
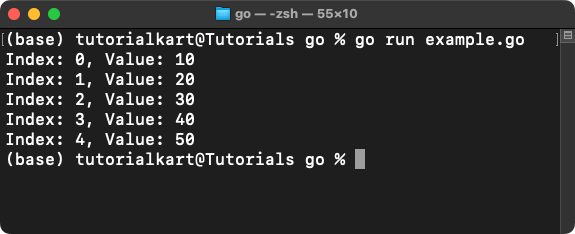
Example 2: Iterating Over a Map
When iterating over a map, the range
keyword returns the key and the value:
package main
import "fmt"
func main() {
countries := map[string]string{
"US": "United States",
"IN": "India",
"JP": "Japan",
}
for key, value := range countries {
fmt.Printf("Key: %s, Value: %s\n", key, value)
}
}
Output
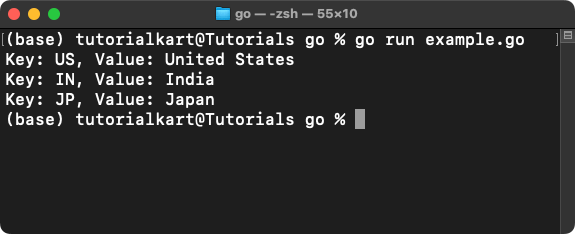
Example 3: Iterating Over a String
When iterating over a string, the range
keyword returns the index and the Unicode code point of each character:
package main
import "fmt"
func main() {
text := "Golang"
for index, char := range text {
fmt.Printf("Index: %d, Character: %c\n", index, char)
}
}
Output
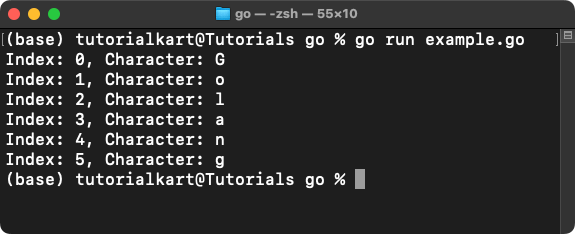
Example 4: Ignoring Index or Value
If you don’t need the index or value, you can use the underscore (_
) to ignore it:
package main
import "fmt"
func main() {
numbers := []int{10, 20, 30, 40, 50}
// Ignore the index
for _, value := range numbers {
fmt.Println("Value:", value)
}
// Ignore the value
for index := range numbers {
fmt.Println("Index:", index)
}
}
Output
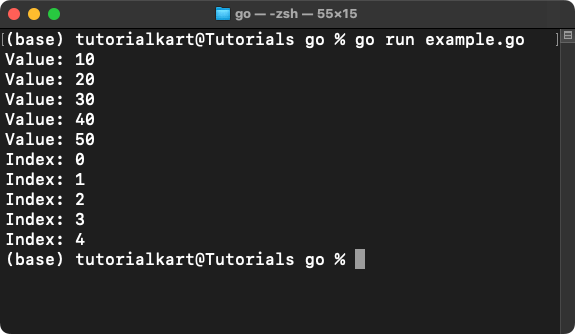
When to Use For Loop Range
- To iterate over arrays or slices for both index and value.
- To traverse maps for keys and values.
- To loop through characters in a string by index and Unicode code points.
- To work with channels for receiving values.
Conclusion
The for
loop with range
in Go provides a concise and efficient way to iterate over collections such as arrays, slices, maps, and strings. Its versatility and simplicity make it a powerful tool for looping through various data structures.