Go – For Loop Range Int
The range
keyword simplifies iteration by providing both the index and the value of each element.
In this tutorial, we will demonstrate how to use the For Loop with the range
keyword to iterate over slices of integers with practical examples and provide detailed explanations.
Iterating Over Slices of Integers
The range
keyword is used to loop through slices and provides two values for each iteration: the index and the corresponding value. Below are examples of iterating through slices of integers.
Example 1: Iterating Over Integers with Index and Value
Here’s an example of iterating through a slice of integers and printing both the index and value:
package main
import "fmt"
func main() {
// Declare a slice of integers
numbers := []int{10, 20, 30, 40, 50}
// Iterate over the slice using range
for index, value := range numbers {
fmt.Printf("Index: %d, Value: %d\n", index, value)
}
}
Explanation
- Declare a Slice: A slice named
numbers
is initialized with integer values. - Use Range: The
range
keyword is used to iterate over the slice, providing the index and value for each element. - Print Index and Value: The index and value are printed using
fmt.Printf
.
Output
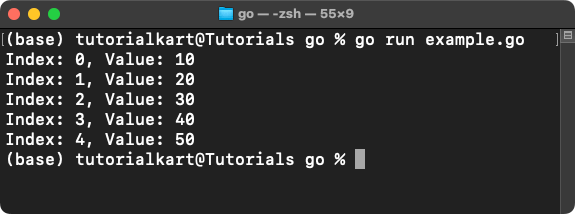
Example 2: Ignoring the Index
If you only need the values, you can ignore the index by using the underscore (_
):
package main
import "fmt"
func main() {
// Declare a slice of integers
numbers := []int{10, 20, 30, 40, 50}
// Iterate over the slice, ignoring the index
for _, value := range numbers {
fmt.Println("Value:", value)
}
}
Explanation
- Declare a Slice: A slice named
numbers
is initialized with integer values. - Use Range: The
range
keyword is used to iterate over the slice. - Ignore the Index: The index is ignored by replacing it with an underscore (
_
), and only the values are printed.
Output
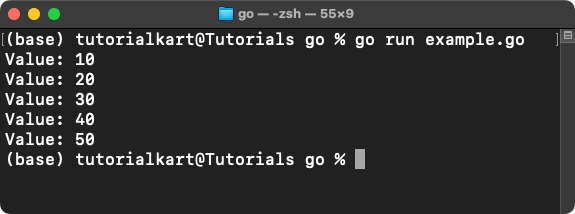
Example 3: Ignoring the Value
If you only need the indices, you can ignore the values:
package main
import "fmt"
func main() {
// Declare a slice of integers
numbers := []int{10, 20, 30, 40, 50}
// Iterate over the slice, ignoring the values
for index := range numbers {
fmt.Println("Index:", index)
}
}
Explanation
- Declare a Slice: A slice named
numbers
is initialized with integer values. - Use Range: The
range
keyword is used to iterate over the slice. - Ignore the Value: The values are ignored by replacing them with an underscore (
_
), and only the indices are printed.
Output
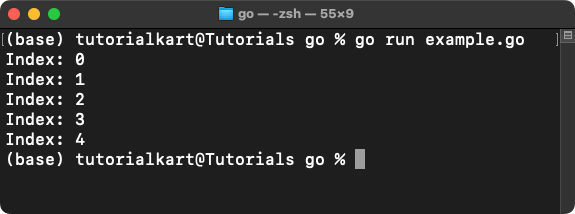
When to Use Range for Integers
You can use range for integer with for loop:
- When you need to process or transform each integer in a slice.
- When you need both the index and the value of elements in a slice.
- When you need to count elements or calculate statistics on integer data.
Conclusion
The for
loop with range
is a powerful tool for iterating over slices of integers in Go. By using or ignoring the index or value as needed, you can efficiently process data in your programs.