Go – For Loop Reverse
Reversing the order of iteration can be useful in many scenarios, such as processing data from the end to the beginning or reversing the traversal of arrays, slices, or strings.
In this tutorial, we will provide examples and detailed explanations on how to use a for loop in Go to iterate through elements in reverse order.
Iterating in Reverse Order Using For Loop
To iterate in reverse order, you can use a standard for
loop and start from the last index of the collection, decrementing the index on each iteration.
Example 1: Reverse Iteration Over a Slice
Let’s iterate through a slice in reverse order:
package main
import "fmt"
func main() {
// Declare a slice
numbers := []int{10, 20, 30, 40, 50}
// Iterate over the slice in reverse order
for i := len(numbers) - 1; i >= 0; i-- {
fmt.Printf("Index: %d, Value: %d\n", i, numbers[i])
}
}
Explanation
- Declare a Slice: A slice named
numbers
is initialized with integer values. - Set Up the For Loop: The loop starts at the last index of the slice using
len(numbers) - 1
and decrements the indexi
on each iteration until it reaches 0. - Access Elements: Each element is accessed using
numbers[i]
. - Print Elements: The index and value of each element are printed in reverse order.
Output
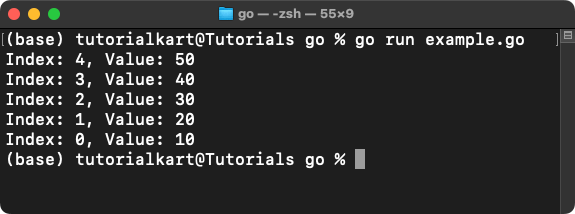
Example 2: Reverse Iteration Over a String
To iterate over a string in reverse order, you can access each character using its index:
package main
import "fmt"
func main() {
// Declare a string
text := "Golang"
// Iterate over the string in reverse order
for i := len(text) - 1; i >= 0; i-- {
fmt.Printf("Index: %d, Character: %c\n", i, text[i])
}
}
Explanation
- Declare a String: A string named
text
is initialized with the value"Golang"
. - Set Up the For Loop: The loop starts at the last index of the string using
len(text) - 1
and decrements the indexi
on each iteration until it reaches 0. - Access Characters: Each character is accessed using
text[i]
. - Print Characters: The index and character are printed in reverse order.
Output
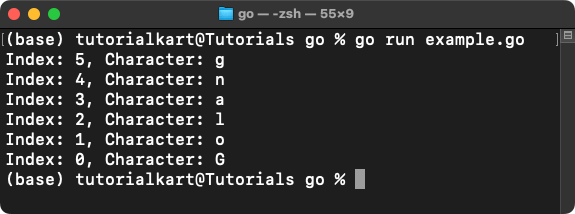
Example 3: Reverse Iteration Over an Array
Here’s an example of iterating over an array in reverse order:
package main
import "fmt"
func main() {
// Declare an array
arr := [5]int{100, 200, 300, 400, 500}
// Iterate over the array in reverse order
for i := len(arr) - 1; i >= 0; i-- {
fmt.Printf("Index: %d, Value: %d\n", i, arr[i])
}
}
Explanation
- Declare an Array: An array named
arr
is initialized with integer values. - Set Up the For Loop: The loop starts at the last index of the array using
len(arr) - 1
and decrements the indexi
on each iteration until it reaches 0. - Access Elements: Each element is accessed using
arr[i]
. - Print Elements: The index and value of each element are printed in reverse order.
Output
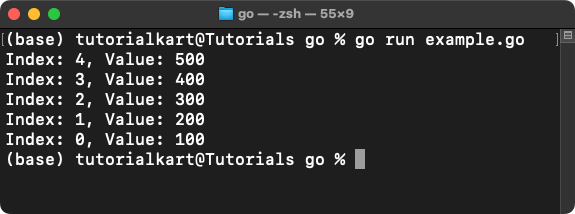
When to Use Reverse Iteration with For Loop
You can use reverse iteration using a For loop:
- To process elements in reverse order (e.g., reversing an array or string).
- To compare elements from the end of a collection to the beginning.
- To handle specific tasks where reverse traversal is more efficient or logical.
Conclusion
Iterating in reverse order using a for
loop in Go is simple and effective. By starting from the last index and decrementing the loop counter, you can traverse arrays, slices, or strings in reverse. This technique is useful in a variety of programming scenarios, making it an essential tool for Go developers.